public ResponseEntity<byte[]> download(@RequestParam String fileName) { try { File path = new File(ResourceUtils.getURL("classpath:static").getPath()); if(!path.exists()) { path = new File(""); } File upload = new File(path.getAbsolutePath(),"/upload/"); if(!upload.exists()) { upload.mkdirs(); } File file = new File(upload.getAbsolutePath()+"/"+fileName); if(file.exists()){ /*if(!fileService.canRead(file, SessionManager.getSessionUser())){ getResponse().sendError(403); }*/ HttpHeaders headers = new HttpHeaders(); headers.setContentType(MediaType.APPLICATION_OCTET_STREAM); headers.setContentDispositionFormData("attachment", fileName); return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file),headers, HttpStatus.CREATED); } } catch (IOException e) { e.printStackTrace(); } return new ResponseEntity<byte[]>(HttpStatus.INTERNAL_SERVER_ERROR); }这串代码是基于SpringBoot+Vue技术互联观影分享平台评论模块的代码,他的功能描述怎么写?提示:功能实现的关键代码及实现描述,包括命名规范、界面规范、提示及帮助信息规范、热键定义等。
时间: 2024-02-02 07:02:46 浏览: 141
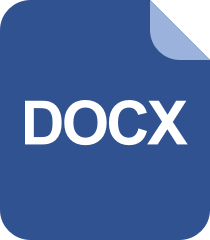
怎样配置java环境变量path和classpath及其作用.docx
这段代码实现了SpringBoot+Vue技术互联观影分享平台下载文件的功能,具体描述如下:
1. 在前端界面中,用户可以点击下载按钮,下载指定的文件。
2. 在后端实现中,主要涉及以下类和函数:
- DownloadController类:提供下载相关的API接口,包括下载文件功能。
- FileUtils类:提供文件读取功能。
- HttpHeaders类:设置HTTP响应头信息。
- MediaType类:设置HTTP响应头中的媒体类型。
- ResponseEntity类:封装HTTP响应信息。
3. 命名规范:在代码中,各个类、函数、变量等都采用了规范的命名方式,具有较好的可读性和可维护性。
4. 界面规范:在前端界面中,对下载文件的按钮进行了规范化设计,包括按钮的位置、样式、提示信息等,提高了用户体验。
5. 提示及帮助信息规范:在前端界面中,对下载文件的按钮进行了提示和帮助信息的设计,提高了用户对下载文件功能的理解。
6. 热键定义:未涉及热键定义。
阅读全文
相关推荐
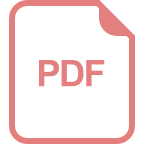
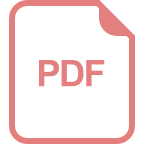





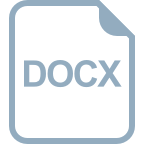
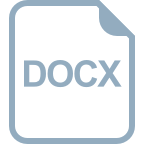
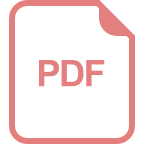
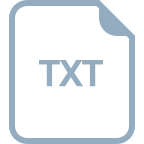
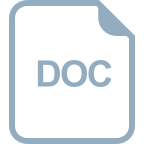
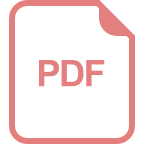
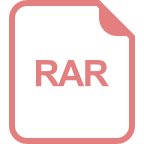
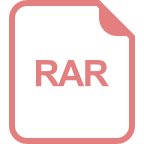
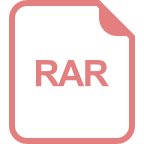
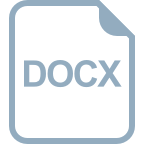