UI创建Python解压压缩包内包含指定字符的文件
时间: 2024-01-06 14:04:08 浏览: 85
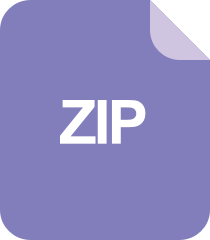
wenshushu:基于 https
这里提供一个使用PyQt5创建UI界面进行解压缩和查找指定文件的示例程序:
```python
import os
import zipfile
from PyQt5.QtWidgets import QApplication, QWidget, QHBoxLayout, QVBoxLayout, QLabel, QLineEdit, QPushButton, QTextEdit, QFileDialog
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 创建界面布局
hbox1 = QHBoxLayout()
hbox1.addWidget(QLabel('Zip文件路径:'))
self.zip_path_edit = QLineEdit()
hbox1.addWidget(self.zip_path_edit)
self.choose_zip_btn = QPushButton('选择')
self.choose_zip_btn.clicked.connect(self.choose_zip_file)
hbox1.addWidget(self.choose_zip_btn)
hbox2 = QHBoxLayout()
hbox2.addWidget(QLabel('解压缩到:'))
self.extract_folder_edit = QLineEdit()
hbox2.addWidget(self.extract_folder_edit)
self.choose_extract_folder_btn = QPushButton('选择')
self.choose_extract_folder_btn.clicked.connect(self.choose_extract_folder)
hbox2.addWidget(self.choose_extract_folder_btn)
hbox3 = QHBoxLayout()
hbox3.addWidget(QLabel('查找文件名中包含:'))
self.search_string_edit = QLineEdit()
hbox3.addWidget(self.search_string_edit)
self.search_btn = QPushButton('查找')
self.search_btn.clicked.connect(self.search_files)
hbox3.addWidget(self.search_btn)
self.result_edit = QTextEdit()
vbox = QVBoxLayout()
vbox.addLayout(hbox1)
vbox.addLayout(hbox2)
vbox.addLayout(hbox3)
vbox.addWidget(self.result_edit)
self.setLayout(vbox)
self.setWindowTitle('Zip文件解压缩和查找')
self.show()
def choose_zip_file(self):
# 选择Zip文件
file_name, _ = QFileDialog.getOpenFileName(self, '选择Zip文件', '', 'Zip文件 (*.zip)')
if file_name:
self.zip_path_edit.setText(file_name)
def choose_extract_folder(self):
# 选择解压缩文件夹
folder_name = QFileDialog.getExistingDirectory(self, '选择解压缩文件夹')
if folder_name:
self.extract_folder_edit.setText(folder_name)
def search_files(self):
zip_file_path = self.zip_path_edit.text()
extract_folder = self.extract_folder_edit.text()
search_string = self.search_string_edit.text()
# 验证输入的路径和字符是否合法
if not os.path.isfile(zip_file_path):
self.result_edit.append('Zip文件路径无效')
return
if not os.path.isdir(extract_folder):
self.result_edit.append('解压缩文件夹路径无效')
return
if not search_string:
self.result_edit.append('请输入要查找的字符串')
return
# 打开Zip文件并解压缩
with zipfile.ZipFile(zip_file_path, 'r') as zip_file:
zip_file.extractall(extract_folder)
self.result_edit.append('Zip文件已解压缩到: ' + extract_folder)
# 遍历Zip文件内的所有文件
for file_name in zip_file.namelist():
# 如果文件名中包含指定字符
if search_string in file_name:
# 打开文件并读取内容
with zip_file.open(file_name) as file:
content = file.read()
# 将文件内容显示在界面上
self.result_edit.append('在文件 ' + file_name + ' 中找到字符串:')
self.result_edit.append(content.decode())
def closeEvent(self, event):
# 窗口关闭时清除解压缩文件夹
extract_folder = self.extract_folder_edit.text()
if os.path.isdir(extract_folder):
for file_name in os.listdir(extract_folder):
file_path = os.path.join(extract_folder, file_name)
if os.path.isfile(file_path):
os.remove(file_path)
elif os.path.isdir(file_path):
os.rmdir(file_path)
os.rmdir(extract_folder)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
app.exec_()
```
该程序使用了PyQt5来创建UI界面,并提供了选择Zip文件、选择解压缩文件夹和查找指定字符的功能。在解压缩Zip文件时,程序会将文件解压缩到指定文件夹中,然后遍历Zip文件内的所有文件,查找文件名中包含指定字符的文件,并显示文件内容。程序还会在窗口关闭时清除解压缩文件夹。
阅读全文
相关推荐
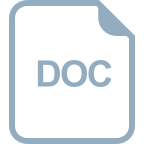
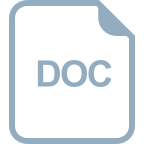
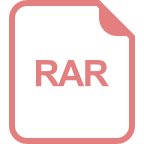
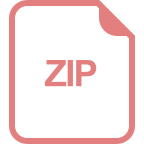
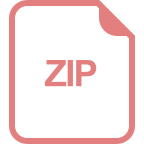
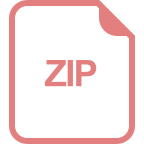
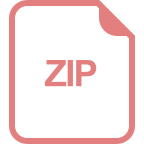
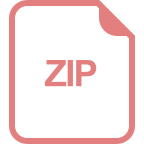
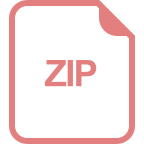
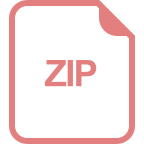
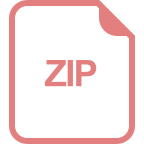
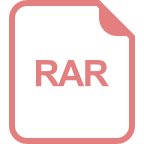
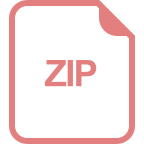
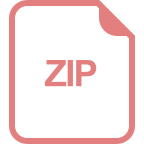
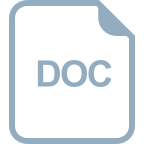
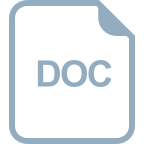
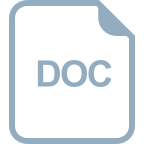
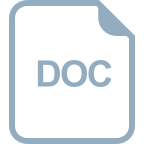