导入导出service层实现类方法的java代码
时间: 2024-06-08 18:10:11 浏览: 98
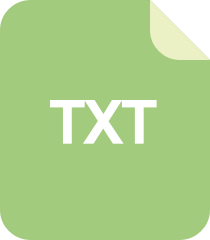
Java导入导出工具类代码实现
你可以使用Java的反射机制来动态导入和导出service层的实现类方法。下面是一个示例代码:
```java
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
public class ServiceExporter {
// 导出service层实现类的方法
public static List<String> exportMethods(Object service) {
List<String> methodList = new ArrayList<>();
Class<?> clazz = service.getClass();
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
methodList.add(method.getName());
}
return methodList;
}
// 导入service层实现类的方法
public static void importMethods(Object service, List<String> methodList) {
Class<?> clazz = service.getClass();
for (String methodName : methodList) {
try {
Method method = clazz.getDeclaredMethod(methodName);
// 执行方法
method.invoke(service);
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
// 示例的service层实现类
class MyService {
public void method1() {
System.out.println("执行method1");
}
public void method2() {
System.out.println("执行method2");
}
}
public class Main {
public static void main(String[] args) {
MyService myService = new MyService();
// 导出方法列表
List<String> methodList = ServiceExporter.exportMethods(myService);
System.out.println("导出的方法列表:" + methodList);
// 导入方法并执行
ServiceExporter.importMethods(myService, methodList);
}
}
```
在上述示例代码中,ServiceExporter类提供了exportMethods和importMethods两个静态方法。exportMethods方法用于导出给定service对象的所有方法名称,将它们存储在一个字符串列表中。importMethods方法根据给定的方法列表,使用反射机制从service对象中获取对应的Method对象,并执行方法。
在示例的Main类中,我们创建了一个MyService对象,并使用ServiceExporter导出了MyService类中的所有方法名称。然后再使用ServiceExporter导入这些方法并执行。
请注意,这只是一个简单的示例,具体的实现方式可能会因项目结构和需求的不同而有所差异。
阅读全文
相关推荐
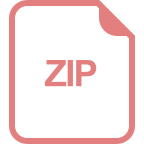
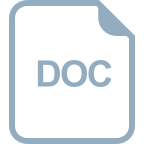
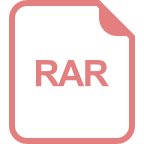
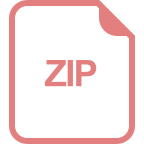
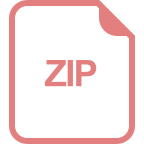
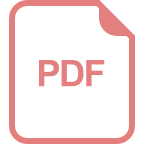
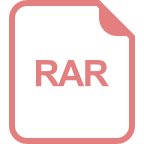
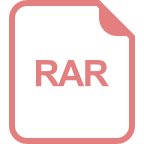
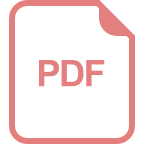
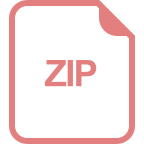
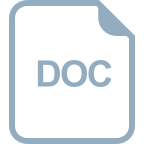
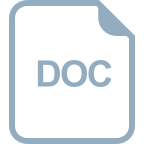
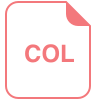
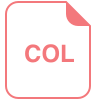
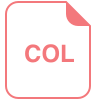

