pic_info.pDataBuf = new unsigned char[1024]; 如何赋值全部为a字符?
时间: 2024-11-09 17:29:12 浏览: 4
在 C++ 中,要将 `pic_info.pDataBuf` 指向的内存区域初始化为全 'a' 字符,你可以遍历整个数组并逐个设置为 'a'。这里假设 `pic_info.pDataBuf` 是一个指向 `unsigned char` 数组的指针,长度为 1024。
```cpp
for (size_t i = 0; i < 1024; ++i) {
pic_info.pDataBuf[i] = 'a';
}
```
这段代码会将从 `pic_info.pDataBuf[0]` 到 `pic_info.pDataBuf[1023]` 的所有位置都设置为字符 'a'。如果你使用的是 C++17 或更高版本,还可以使用范围-based for 循环简化:
```cpp
pic_info.pDataBuf = '\0'; // 初始化第一个元素为'\0'
std::fill(pic_info.pDataBuf, pic_info.pDataBuf + 1024, 'a');
```
这里我们先用 `\0` 初始化第一个元素(通常用于字符串结束标记),然后使用 `std::fill` 函数填充剩余的元素。
相关问题
帮我写一段c++代码,具体的功能为:读取pbuf中取数据到pDataBuf中,pDataBuf大小为64*1024,循环读取,直到读完
好的,以下是一个示例代码,可以帮助您实现上述功能:
```c++
#include <iostream>
#include <cstring>
// 从pbuf中读取数据到pDataBuf中,直到读取完毕
void readData(char* pDataBuf, char* pbuf) {
int totalBytesRead = 0;
int bytesRead = 0;
while (totalBytesRead < 64*1024) {
bytesRead = std::min((int)strlen(pbuf), 64*1024 - totalBytesRead); // 取pbuf和pDataBuf剩余空间大小的最小值
if (bytesRead == 0) {
break; // 如果读取完毕,则跳出循环
}
memcpy(pDataBuf + totalBytesRead, pbuf, bytesRead); // 将pbuf中的数据复制到pDataBuf中
pbuf += bytesRead;
totalBytesRead += bytesRead;
}
}
int main() {
char pDataBuf[64*1024]; // 存储数据的缓冲区
char pbuf[] = "Hello, World!"; // 数据来源
readData(pDataBuf, pbuf);
std::cout << pDataBuf << std::endl; // 输出读取到的数据
return 0;
}
```
上述代码中,我们使用了一个名为readData的函数来实现数据的读取。在该函数中,我们使用一个循环来读取数据,每次读取的数据存储到pDataBuf + totalBytesRead的位置,totalBytesRead表示已经读取的字节数。在每次循环中,我们使用min函数来计算还需要读取的字节数,以确保不会超出pDataBuf的范围。如果已经读取完毕,则跳出循环。最后,我们在主函数中调用readData函数,并输出读取到的数据。
希望这个示例代码能够对您有所帮助。如果您还有其他问题或者需要进一步解释,请随时提出。
pDataBuf大小为64*1024;从另一个buffer中读取pbuf中取数据到pDataBuf,循环读取,直到读完
好的,您的问题是如何循环读取另一个buffer中的数据并将其存储到pDataBuf中,直到读取完毕。我可以给您提供一个参考代码,具体实现可能需要根据您的具体情况进行调整:
```
int totalBytesRead = 0;
int bytesRead = 0;
while (totalBytesRead < 64*1024) {
bytesRead = readFromOtherBuffer(pbuf, pDataBuf + totalBytesRead, 64*1024 - totalBytesRead);
if (bytesRead == 0) {
break; // 如果读取完毕,则跳出循环
}
totalBytesRead += bytesRead;
}
```
上述代码中,我们使用一个循环来读取数据,每次读取的数据存储到pDataBuf + totalBytesRead的位置,totalBytesRead表示已经读取的字节数。readFromOtherBuffer是一个用于从另一个buffer中读取数据的函数,该函数的返回值为实际读取的字节数。当totalBytesRead等于64*1024时,表示已经读取了足够的数据,可以跳出循环。如果readFromOtherBuffer返回0,表示已经读取完毕,也可以跳出循环。
希望这个代码能够对您有所帮助。如果您还有其他问题或者需要进一步解释,请随时提出。
阅读全文
相关推荐
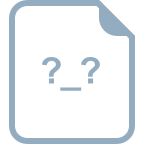
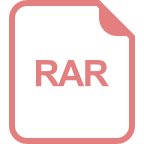
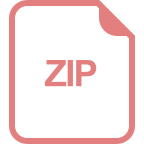
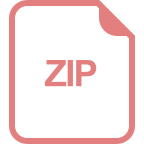
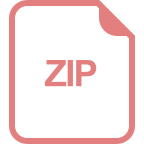
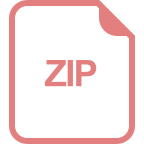
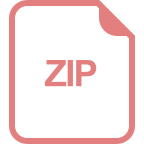
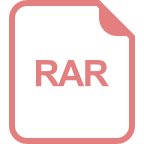
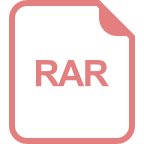