设计一个学生类char*name,double score给出数组并求数组中的最高分和最低分c++
时间: 2024-11-11 10:21:53 浏览: 7
设计一个名为`Student`的C++类,用于存储学生的姓名(name)和分数(score),你可以这样做:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Student {
public:
// 构造函数
Student(const char* name, double score)
: name(name), score(score) {}
// 获取名字
const char* getName() const { return name; }
// 获取分数
double getScore() const { return score; }
private:
// 学生姓名
const char* name;
// 学生成绩
double score;
};
// 函数模板求解向量中的最高分和最低分
template<typename T>
pair<T, T> findMinMax(const vector<T>& scores) {
if (scores.empty()) {
throw invalid_argument("The vector is empty");
}
T min = scores[0];
T max = scores[0];
for (const auto& s : scores) {
if (s < min) {
min = s;
} else if (s > max) {
max = s;
}
}
return make_pair(min, max);
}
int main() {
// 示例学生数据
Student students[] = {
{"张三", 85.0},
{"李四", 92.0},
{"王五", 78.0}
};
// 存储分数的向量
vector<double> studentScores;
for (const auto& student : students) {
studentScores.push_back(student.getScore());
}
// 求解最高分和最低分
pair<double, double> result = findMinMax(studentScores);
cout << "最高分: " << result.first << ", 最低分: " << result.second << endl;
return 0;
}
```
在这个例子中,我们首先定义了一个`Student`类,然后创建一个`findMinMax`函数模板,它接受一个`T`类型的向量作为输入,并返回一对`min`和`max`值。在`main`函数中,我们将学生的分数添加到`studentScores`向量中,最后调用`findMinMax`计算最高分和最低分。
阅读全文
相关推荐
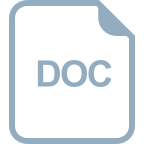
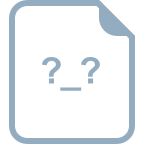
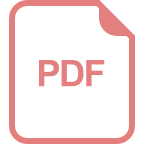
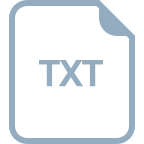
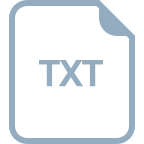
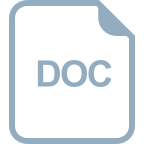
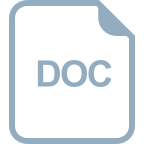
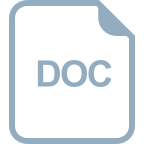
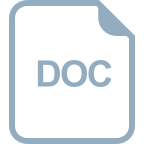








