c语言编写:【问题描述】求一个n个数列表的第k最小元素 【输入形式】数列表长度n、第k和列表 【输出形式】第k最小的元素 【样例输入】9 5 4 1 10 8 7 12 9 2 15 【样例输出】8
时间: 2024-10-08 14:20:58 浏览: 45
在C语言中,可以使用一种叫做“快速选择”(QuickSelect)算法或者结合“堆”数据结构来求解这个问题。这里提供一个基于快速选择的基本思路:
```c
#include <stdio.h>
// 交换两个数的值
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
// 随机选择pivot
int random Pivot(int arr[], int low, int high) {
srand(time(0));
return arr[low + rand() % (high - low + 1)];
}
// 快速选择
int quickSelect(int arr[], int low, int high, int k) {
if (low == high)
return arr[low];
int pivotIndex = partition(arr, low, high);
if (k == pivotIndex)
return arr[k];
else if (k < pivotIndex)
return quickSelect(arr, low, pivotIndex - 1, k);
else
return quickSelect(arr, pivotIndex + 1, high, k);
}
// 对数组进行分区操作,返回pivot的新位置
int partition(int arr[], int low, int high) {
int pivotValue = random Pivot(arr, low, high);
int i = (low - 1); // Index of smaller element
for (int j = low; j <= high - 1; j++) {
// 如果当前元素小于pivot,则交换并增加i
if (arr[j] < pivotValue) {
i++;
swap(&arr[i], &arr[j]);
}
}
// 将pivot放到正确的位置
swap(&arr[i + 1], &arr[high]);
return i + 1;
}
int main() {
int n, k;
scanf("%d %d", &n, &k);
int arr[n];
for (int i = 0; i < n; i++) {
scanf("%d", &arr[i]);
}
int kthSmallest = quickSelect(arr, 0, n - 1, k - 1); // 函数索引减1是因为我们从0开始计数
printf("The %dth smallest element is: %d\n", k, kthSmallest);
return 0;
}
```
这个程序首先读取输入的列表,然后通过快速选择找到第k小的元素。注意,`quickSelect`函数内部的`partition`函数用于将数组划分为两部分,左边的部分都比pivot小,右边的部分都大于等于pivot。
阅读全文
相关推荐
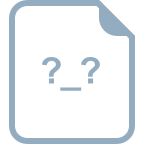
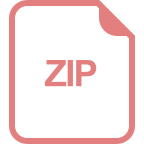
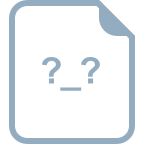
















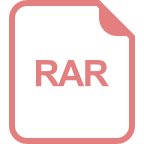