C语言用链表实现:将字符串t复制到字符串s的末端,并且返回新的字符串的首地址,并编写主程序输出。
时间: 2024-12-08 13:15:45 浏览: 35
在C语言中,你可以使用链表数据结构来实现这个功能。这里是一个简单的例子,首先定义一个字符链表节点(`struct Node`),然后创建一个函数`appendString`来完成字符串的复制并连接:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表节点结构
typedef struct Node {
char data;
struct Node* next;
} Node;
// 函数声明
Node* appendString(Node* head, const char* t);
// 主函数
int main() {
// 初始化头节点
Node* head = (Node*)malloc(sizeof(Node));
head->data = '\0'; // 首个节点初始化为空字符
head->next = NULL; // 初始化下一个指针
// 待复制的字符串t
char str_t[] = "Hello, World!";
// 使用appendString函数复制并连接
Node* new_head = appendString(head, str_t);
// 输出新字符串
printf("New string: ");
while (new_head != NULL) {
printf("%c", new_head->data);
new_head = new_head->next;
}
printf("\n");
// 释放内存
free(head); // 可能需要额外处理free(new_head),取决于new_head是否指向了分配的内存
return 0;
}
// 实现字符串复制并连接的函数
Node* appendString(Node* head, const char* t) {
if (*t == '\0') { // 如果t已经结束,直接返回当前链表的头结点
return head;
}
// 创建一个新的节点,存储t的第一个字符
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = *t;
newNode->next = head;
// 继续处理剩余的t
return appendString(newNode->next, t + 1);
}
```
这个程序会把字符串`t`追加到`s`的末尾,如果`s`为空,则直接复制`t`;否则,它会在`s`的最后一个字符后面插入`t`的第一个字符,然后递归处理`t`剩余部分。
阅读全文
相关推荐
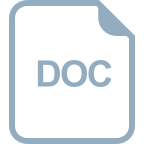
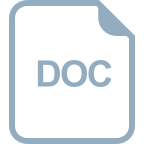
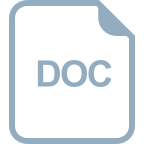
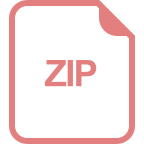
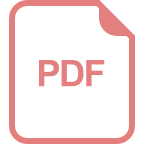
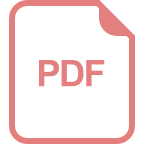
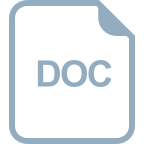
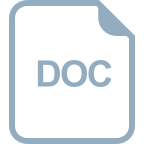
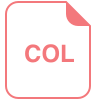
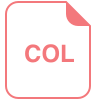
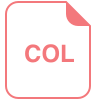
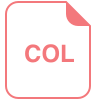
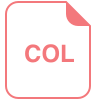
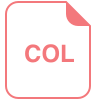
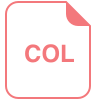
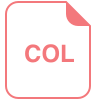
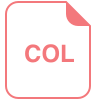
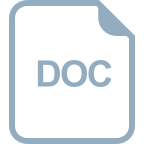
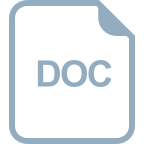