C#中去除字符串空格的函数
时间: 2024-05-03 20:17:41 浏览: 96
在C#中,可以使用`Trim()`函数去除字符串的前后空格,使用`Replace()`函数去除字符串中所有空格。
例如,假设有一个字符串`str`,可以通过以下代码去除字符串中所有空格:
```
str = str.Replace(" ", "");
```
如果只需要去除字符串的前后空格,可以使用以下代码:
```
str = str.Trim();
```
这两个函数都会返回一个新的字符串,原始字符串不会被修改。
相关问题
C# 去掉字符串中的空格
在 C# 中,如果你想去掉字符串中的所有空格,可以使用 `Replace` 或 `Trim` 方法结合 `StringSplitOptions.RemoveEmptyEntries` 参数。以下是两种常见的方式:
1. 使用 `Replace` 方法:
```csharp
string str = "Hello World";
str = str.Replace(" ", "");
```
这会将所有的空格替换为空字符,结果为 "HelloWorld"。
2. 使用 `Trim` 和 `RemoveEmptyEntries`:
```csharp
string str = " Hello World ";
str = str.Trim(new char[] { ' ', '\t', '\r', '\n' }, StringSplitOptions.RemoveEmptyEntries);
```
这里 `Trim` 函数移除字符串开头和结尾的空白字符,而 `StringSplitOptions.RemoveEmptyEntries` 表示如果连续的空项存在则全部删除,结果也是 "HelloWorld"。
c#中字符串函数大全
在C#中,字符串是一种特殊的数据类型,其内置了许多方便处理文本的函数。以下是一些常用的字符串函数示例:
1. `string.Length` 或 `.Length`: 返回字符串的字符数。
```csharp
string str = "Hello";
int length = str.Length; // length will be 5
```
2. `string.IsNullOrEmpty()` 或 `.IsNullOrWhiteSpace()`: 检查字符串是否为空或只包含空格。
```csharp
string str;
if (string.IsNullOrEmpty(str)) {
Console.WriteLine("String is null or empty");
}
```
3. `string.Concat()`: 连接两个或更多字符串。
```csharp
string combinedStr = string.Concat("Hello", " ", "World"); // combinedStr will be "Hello World"
```
4. `string.Substring()` 或 `string.Slice()`: 提取子串。
```csharp
string str = "HelloWorld";
string subStr = str.Substring(6); // subStr will be "World"
```
5. `string.IndexOf()` 或 `IndexOf(string, int)`: 查找指定字符或子串的位置。
```csharp
int index = str.IndexOf('W'); // index will be 6
```
6. `string.Replace()` 或 `Replace(oldValue, newValue)`: 替换字符串中的某个部分。
```csharp
string newStr = str.Replace("World", "Universe"); // newStr will be "Hello Universe"
```
7. `string.Split()` 或 `Split(char[])`: 将字符串分割成子字符串数组。
```csharp
string[] words = str.Split(' '); // words will contain ["Hello", "World"]
```
8. `string.Trim()` 或 `TrimStart()`、`TrimEnd()`: 移除字符串两端的空白字符。
```csharp
string trimmedStr = str.Trim(); // trimmedStr will remove leading and trailing spaces
```
还有很多其他函数,如格式化字符串(`string.Format()`)、正则表达式匹配(`Regex*`类)等。
阅读全文
相关推荐
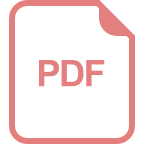
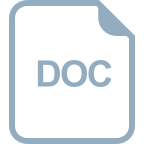
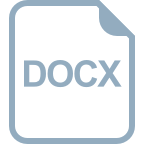
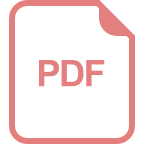
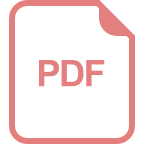
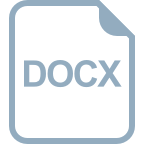
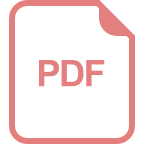
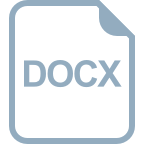
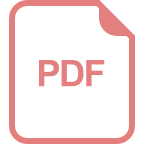
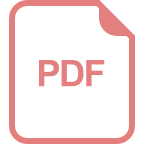
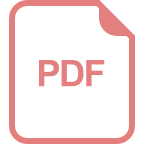
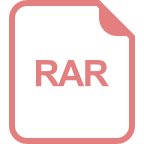
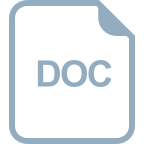
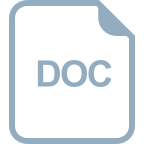
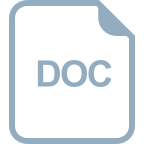