python绘制词云图并展示
时间: 2023-06-21 18:12:38 浏览: 118
好的,下面是一个简单的Python代码,用于生成并展示词云。
首先,需要安装 Python 的词云生成库 `wordcloud` 和图片处理库 `PIL`。可以使用以下命令进行安装:
```
pip install wordcloud
pip install Pillow
```
然后,我们需要读取一段文本,并对其中的单词进行统计。这里以文本文件 `example.txt` 为例:
```
This is an example text. It contains multiple words and some of them are repeated. This text is used for generating a word cloud.
```
我们可以使用 Python 的 `collections` 模块中的 `Counter` 类来统计单词出现的次数,然后把结果传递给 `wordcloud` 库来生成词云。下面是完整的代码:
```python
from collections import Counter
from wordcloud import WordCloud
from PIL import Image
import numpy as np
# 读取文本文件
with open("example.txt") as f:
text = f.read()
# 统计单词出现的次数
words_count = Counter(text.split())
# 生成词云
wordcloud = WordCloud(background_color="white").generate_from_frequencies(words_count)
# 读取图片作为背景
mask = np.array(Image.open("mask.png"))
# 把词云图片和背景图片合并
wordcloud_image = WordCloud(background_color="white", mask=mask).generate_from_frequencies(words_count)
image_colors = ImageColorGenerator(mask)
wordcloud_image = wordcloud_image.recolor(color_func=image_colors)
# 显示词云
import matplotlib.pyplot as plt
plt.imshow(wordcloud_image, interpolation="bilinear")
plt.axis("off")
plt.show()
```
其中,`WordCloud()` 函数可以接受一些参数,例如 `background_color` 表示背景色,`mask` 表示词云的形状,这里使用了一张名为 `mask.png` 的图片作为背景。词云生成后,还可以使用 `ImageColorGenerator()` 函数将背景颜色应用到词云上。
最后,使用 `matplotlib` 库中的 `imshow()` 函数来显示词云。运行代码后,会弹出一个窗口展示生成的词云图像。
注意:在生成词云之前,需要确保文本文件和背景图片都存在,并且路径正确。
阅读全文
相关推荐
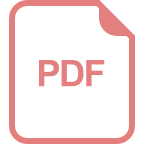
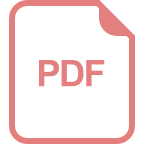







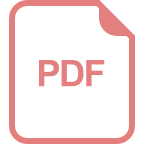
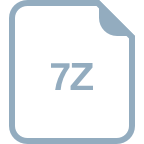
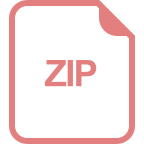
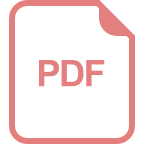
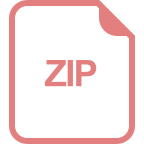
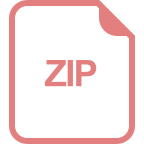