编写一个c语言程序,从一个文件中读入单词(即:以空格分隔的字符串),并对单词进行排序,删除重复出现的单词,然后将结果输出到另一个文件中。 【输入形式】 从一个文件sort.in中读入单词。 对单词进行排序,删除重复出现的单词,然后将结果输出到文件sort.out中。
时间: 2024-02-25 19:53:47 浏览: 24
好的,这是一个文件操作和字符串操作的问题,我来帮您解析一下。
首先,我们需要打开输入文件和输出文件。然后,我们可以使用fgets函数读取输入文件中的每一个单词,并存储到一个字符串数组中。接着,我们可以使用qsort函数对字符串数组进行排序,然后删除重复出现的单词。
最后,我们可以使用fprintf函数将结果输出到输出文件中,然后关闭输入文件和输出文件。
下面是一个初步的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 100
#define MAX_WORDS 1000
int cmp(const void *a, const void *b) {
return strcmp(*(const char **)a, *(const char **)b);
}
int main() {
FILE *input_file = fopen("sort.in", "r");
FILE *output_file = fopen("sort.out", "w");
char words[MAX_WORDS][MAX_WORD_LEN];
int word_count = 0;
// 读取输入文件中的单词
char line[MAX_WORD_LEN];
while (fgets(line, MAX_WORD_LEN, input_file) != NULL) {
char *word = strtok(line, " \t\n");
while (word != NULL) {
strcpy(words[word_count++], word);
word = strtok(NULL, " \t\n");
}
}
// 对单词进行排序
qsort(words, word_count, sizeof(words[0]), cmp);
// 删除重复出现的单词
int unique_count = 0;
for (int i = 0; i < word_count; i++) {
if (i == 0 || strcmp(words[i], words[i-1]) != 0) {
strcpy(words[unique_count++], words[i]);
}
}
// 输出结果到输出文件中
for (int i = 0; i < unique_count; i++) {
fprintf(output_file, "%s ", words[i]);
}
// 关闭文件
fclose(input_file);
fclose(output_file);
return 0;
}
```
当然,这只是一个初步的代码示例,您可以根据自己的需求进行适当的修改。
相关推荐
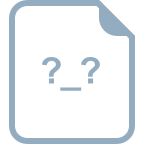
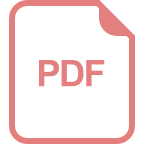
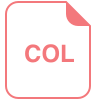
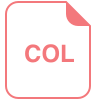
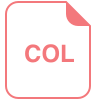
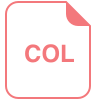
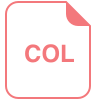









