字符串处理:C语言中的字符串操作和常见问题解决
发布时间: 2023-12-17 02:03:50 阅读量: 13 订阅数: 11 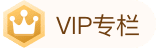
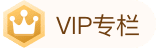
# 章节一:C语言中的字符串处理基础
C语言中的字符串处理是编程中常见的任务之一,了解字符串的基础知识对于进行有效的编程非常重要。本章将介绍C语言中字符串处理的基础知识,包括字符串的定义和表示、字符串常量与字符数组的区别、字符串的输入和输出方式,以及字符串长度和空字符的重要性。让我们一起来深入了解!
## 2. 章节二:常见的字符串操作函数
在C语言中,有许多内置的字符串操作函数可用于处理和操纵字符串。这些函数提供了对字符串的复制、连接、比较、查找等操作。在本章节中,我们将介绍几个常见的字符串操作函数及其使用方法。
### 2.1 strcpy()和strncpy()函数
#### strcpy()
`strcpy()`函数用于将一个字符串复制到另一个字符串中。它的原型如下:
```c
char* strcpy(char* destination, const char* source);
```
其中,`destination`是要复制到的目标字符串,`source`是要复制的源字符串。`strcpy()`函数会将`source`字符串中的字符复制到`destination`字符串中,直到遇到空字符`\0`为止。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, world!";
char destination[20];
strcpy(destination, source);
printf("Destination string: %s\n", destination);
return 0;
}
```
输出结果:
```
Destination string: Hello, world!
```
#### strncpy()
`strncpy()`函数与`strcpy()`函数类似,也用于将一个字符串复制到另一个字符串中。但是,`strncpy()`函数可以指定要复制的字符数目,以防目标字符串溢出。它的原型如下:
```c
char* strncpy(char* destination, const char* source, size_t num);
```
其中,`destination`是要复制到的目标字符串,`source`是要复制的源字符串,`num`是要复制的字符数。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, world!";
char destination[10];
strncpy(destination, source, 5);
destination[5] = '\0'; // 手动添加空字符,避免输出超过目标字符数
printf("Destination string: %s\n", destination);
return 0;
}
```
输出结果:
```
Destination string: Hello
```
### 2.2 strcat()和strncat()函数
#### strcat()
`strcat()`函数用于将一个字符串连接到另一个字符串的末尾。它的原型如下:
```c
char* strcat(char* destination, const char* source);
```
其中,`destination`是目标字符串,`source`是要连接的源字符串。`strcat()`函数会将`source`字符串中的字符追加到`destination`字符串的末尾,并在最后添加一个空字符`\0`。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char destination[20] = "Hello";
char source[] = ", world!";
strcat(destination, source);
printf("Destination string: %s\n", destination);
return 0;
}
```
输出结果:
```
Destination string: Hello, world!
```
#### strncat()
`strncat()`函数与`strcat()`函数类似,也用于将一个字符串连接到另一个字符串的末尾。但是,`strncat()`函数可以指定要连接的字符数目,以防目标字符串溢出。它的原型如下:
```c
char* strncat(char* destination, const char* source, size_t num);
```
其中,`destination`是目标字符串,`source`是要连接的源字符串,`num`是要连接的字符数。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char destination[20] = "Hello";
char source[] = ", world!";
strncat(destination, source, 5);
destination[11] = '\0'; // 手动添加空字符,避免输出超过目标字符数
printf("Destination string: %s\n", destination);
return 0;
}
```
输出结果:
```
Destination string: Hello, w
```
### 2.3 strcmp()和strncmp()函数
#### strcmp()
`strcmp()`函数用于比较两个字符串是否相等。它的原型如下:
```c
int strcmp(const char* str1, const char* str2);
```
其中,`str1`和`str2`是要比较的两个字符串。如果两个字符串相等,则返回值为0;如果第一个字符串小于第二个字符串,则返回值小于0;如果第一个字符串大于第二个字符串,则返回值大于0。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello";
char str2[] = "Hello";
if (strcmp(str1, str2) == 0) {
printf("The two strings are equal.\n");
} else {
printf("The two strings are not equal.\n");
}
return 0;
}
```
输出结果:
```
The two strings are equal.
```
#### strncmp()
`strncmp()`函数与`strcmp()`函数类似,也用于比较两个字符串是否相等。但是,`strncmp()`函数可以指定要比较的字符数目,以防止出现不必要的比较和溢出。它的原型如下:
```c
int strncmp(const char* str1, const char* str2, size_t num);
```
其中,`str1`和`str2`是要比较的两个字符串,`num`是要比较的字符数。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello, world!";
char str2[] = "Hello";
if (strncmp(str1, str2, 5) == 0) {
printf("The two strings are equal.\n");
} else {
printf("The two strings a
```
0
0
相关推荐
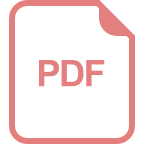
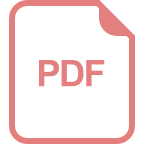





