TypeError TypeError: argument 'hashed_password': 'str' object cannot be converted to 'PyBytes'修改
时间: 2024-11-25 19:01:02 浏览: 27
在代码中出现 `TypeError: argument 'hashed_password': 'str' object cannot be converted to 'PyBytes'` 错误的原因是 `bcrypt.hashpw` 函数期望第二个参数是一个字节字符串(`bytes`),而你传入的是一个普通的字符串(`str`)。你需要将字符串转换为字节字符串。
具体来说,在 `register` 函数中的以下部分:
```python
hashed_password = bcrypt.hashpw(password.encode('utf-8'), bcrypt.gensalt())
try:
cursor.execute('INSERT INTO users (username, password) VALUES (?, ?)', (username, hashed_password))
```
`hashed_password` 是一个字节字符串,但 `cursor.execute` 方法期望所有参数都是普通字符串。因此,需要将 `hashed_password` 转换为普通字符串。可以使用 `hashed_password.decode('utf-8')` 来实现这一点。
修改后的代码如下:
```python
hashed_password = bcrypt.hashpw(password.encode('utf-8'), bcrypt.gensalt()).decode('utf-8')
try:
cursor.execute('INSERT INTO users (username, password) VALUES (?, ?)', (username, hashed_password))
```
同样地,在 `login` 函数中也需要进行类似的处理:
```python
cursor.execute('SELECT id, password FROM users WHERE username = ?', (username,))
result = cursor.fetchone()
if result and bcrypt.checkpw(password.encode('utf-8'), result[1].encode('utf-8')):
```
这里,`result[1]` 是从数据库中取出的哈希密码,它是一个普通字符串,需要转换为字节字符串才能传递给 `bcrypt.checkpw` 函数。
完整修改后的相关部分代码如下:
```python
@app.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
conn = connect_db()
cursor = conn.cursor()
hashed_password = bcrypt.hashpw(password.encode('utf-8'), bcrypt.gensalt()).decode('utf-8')
try:
cursor.execute('INSERT INTO users (username, password) VALUES (?, ?)', (username, hashed_password))
conn.commit()
flash("注册成功!", 'success')
logging.info("用户 %s 注册成功!", username)
return redirect(url_for('login'))
except sqlite3.IntegrityError:
flash("用户名已存在!", 'warning')
logging.warning("用户名 %s 已存在!", username)
finally:
conn.close()
return render_template('register.html')
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
conn = connect_db()
cursor = conn.cursor()
cursor.execute('SELECT id, password FROM users WHERE username = ?', (username,))
result = cursor.fetchone()
if result and bcrypt.checkpw(password.encode('utf-8'), result[1].encode('utf-8')):
session['user_id'] = result[0]
flash("登录成功!", 'success')
logging.info("用户 %s 登录成功!", username)
return redirect(url_for('dashboard'))
else:
flash("用户名或密码错误!", 'danger')
logging.warning("用户名或密码错误!")
conn.close()
return render_template('login.html')
```
这样修改后,应该可以解决 `TypeError` 问题。
阅读全文
相关推荐
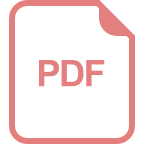
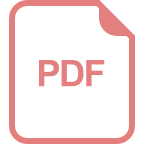
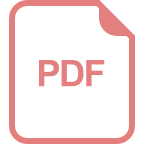















