C# FTP 下载文件
时间: 2024-09-20 13:15:11 浏览: 17
在C#中,通过System.IO.FtpClient库可以方便地实现FTP下载文件的功能。以下是一个简单的示例,展示了如何使用FTPClient类连接到FTP服务器、下载文件:
```csharp
using System;
using System.IO;
using System.Net;
class Program
{
static void Main(string[] args)
{
string ftpServer = "ftp.example.com";
int ftpPort = 21; // 默认端口,也可以自定义
string username = "your_username";
string password = "your_password";
string remoteFilePath = "/path/to/remote/file.txt";
string localFilePath = @"C:\local\file.txt"; // 本地保存路径
try
{
FtpWebRequest request = (FtpWebRequest)WebRequest.Create($"ftp://{ftpServer}:{ftpPort}/{remoteFilePath}");
request.Method = WebRequestMethods.Ftp.DownloadFile;
request.Credentials = new NetworkCredential(username, password);
using (FtpWebResponse response = (FtpWebResponse)request.GetResponse())
{
Stream responseStream = response.GetResponseStream();
using (FileStream fileStream = File.OpenWrite(localFilePath))
{
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = responseStream.Read(buffer, 0, buffer.Length)) > 0)
{
fileStream.Write(buffer, 0, bytesRead);
}
Console.WriteLine("文件下载完成");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"下载失败: {ex.Message}");
}
Console.ReadLine(); // 等待用户按下Enter键后退出
}
}
```
在这个例子中,你需要将`ftpServer`、`username`和`password`替换为实际的FTP服务器地址、用户名和密码。程序会将远程文件从指定路径下载到本地指定位置。
相关推荐
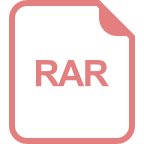
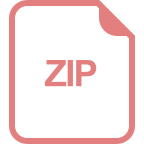
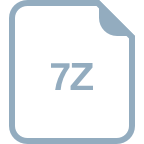














