编写程序以顺序表和链表两种方式实现: (1) 对图书表的创建(初始创建5本书); (2) 分别在第5和第11的位置上插入两本书(011,python程序设计,23)和(012,数据结构,55) (3) 删除第2本书 (4) 获取第3本
时间: 2023-11-20 13:06:28 浏览: 36
很高兴为您解答问题!以下是两种方式实现的程序:
顺序表方式:
```python
class Book:
def __init__(self, id, name, price):
self.id = id
self.name = name
self.price = price
class BookList:
def __init__(self):
self.book_list = [Book(001, "Java程序设计", 45),
Book(002, "Python编程:从入门到实践", 58),
Book(003, "C语言程序设计", 36),
Book(004, "算法导论", 78),
Book(005, "计算机组成原理", 67)]
def insert_book(self, index, book):
self.book_list.insert(index-1, book)
def delete_book(self, index):
self.book_list.pop(index-1)
def get_book(self, index):
return self.book_list[index-1]
if __name__ == "__main__":
book_list = BookList()
book_list.insert_book(5, Book(011, "python程序设计", 23))
book_list.insert_book(11, Book(012, "数据结构", 55))
book_list.delete_book(2)
book = book_list.get_book(3)
print("获取到的第3本书是:", book.name)
```
链表方式:
```python
class Book:
def __init__(self, id, name, price):
self.id = id
self.name = name
self.price = price
self.next = None
class BookList:
def __init__(self):
self.head = None
self.tail = None
self.length = 0
self.create_books()
def create_books(self):
self.head = Book(001, "Java程序设计", 45)
self.tail = self.head
self.tail.next = Book(002, "Python编程:从入门到实践", 58)
self.tail = self.tail.next
self.tail.next = Book(003, "C语言程序设计", 36)
self.tail = self.tail.next
self.tail.next = Book(004, "算法导论", 78)
self.tail = self.tail.next
self.tail.next = Book(005, "计算机组成原理", 67)
self.tail = self.tail.next
def insert_book(self, index, book):
if index == 1:
book.next = self.head
self.head = book
else:
pre = self.head
cur = pre.next
i = 2
while cur and i < index:
pre = cur
cur = cur.next
i += 1
pre.next = book
book.next = cur
def delete_book(self, index):
if index == 1:
self.head = self.head.next
else:
pre = self.head
cur = pre.next
i = 2
while cur and i < index:
pre = cur
cur = cur.next
i += 1
pre.next = cur.next
def get_book(self, index):
cur = self.head
i = 1
while cur and i < index:
cur = cur.next
i += 1
return cur
if __name__ == "__main__":
book_list = BookList()
book_list.insert_book(5, Book(011, "python程序设计", 23))
book_list.insert_book(11, Book(012, "数据结构", 55))
book_list.delete_book(2)
book = book_list.get_book(3)
print("获取到的第3本书是:", book.name)
```
注意:以上程序只是演示如何实现对图书表的操作,实际应用中可能需要更多的功能和处理。
相关推荐
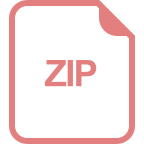















