#include<stdio.h> @-@ int main() { int x,y; scanf("%d%d",&x,&y); printf("Before swap:x=%d,y=%d\n",x,y); swap(&x,&y); printf("After swap:x=%d,y=%d\n",x,y); return 0; }
时间: 2023-11-27 21:05:57 浏览: 79
这段代码中,你使用了 `swap` 函数,但是在 C 语言中并没有内置的 `swap` 函数,所以需要自己实现。可以使用指针来实现两数交换的功能,如下所示:
```c
#include <stdio.h>
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x, y;
printf("Enter two integers: ");
scanf("%d%d", &x, &y);
printf("Before swapping: x = %d, y = %d\n", x, y);
swap(&x, &y);
printf("After swapping: x = %d, y = %d\n", x, y);
return 0;
}
```
在这个程序中,我们定义了一个 `swap` 函数,该函数接收两个整型指针 `a` 和 `b`,并通过指针来交换两个整数的值。在主函数中,我们通过 `scanf` 函数来读入两个整数 `x` 和 `y`,然后调用 `swap` 函数来交换它们的值,并在交换前后分别输出 `x` 和 `y` 的值,以此验证两数交换的顺序是否正确。
你可以将这段代码复制到本地的 C 编译器中运行,也可以在 NBUOJ 上提交这个代码,以验证它的正确性。
相关问题
#include<stdio.h> int main() int x,y scanf('%d" &x) if(x<1) if(1<=x <10) y=x*2-1 if(x>=10) y=x*3-1 printf(" %d",&y) return 0
这段 C 语言代码有一个基本的结构,它试图根据输入的整数 `x` 来计算并打印一个新的值 `y`。然而,代码有几个语法错误和逻辑问题:
1. 在 `main()` 函数声明之前,不需要包含 `stdio.h` 文件头,因为这是标准库文件。
2. `int main()` 需要有花括号 `{}` 包裹其内部的代码块。
3. `scanf` 的格式字符串 `%d` 后面应去掉引号 `"`,正确的是 `%d`。
4. `scanf` 应该有分号 `;` 结束,然后是变量名 `&x`,正确的格式是 `scanf("%d", &x);`
5. 内部的 `if` 语句嵌套不规范,且逻辑判断也不清晰。你需要合并条件判断,并根据 `x` 的范围来设置 `y` 的计算规则。
下面是一个更合理的修订版:
```c
#include <stdio.h>
int main() {
int x, y;
scanf("%d", &x);
if (x >= 1 && x <= 9) { // 将两个条件合并,简化判断
y = x * 2 - 1;
} else if (x >= 10) {
y = x * 3 - 1;
}
printf(" %d", y); // 注意,输出前需要加空格,且末尾无需取地址符 "&"
return 0;
}
```
用指针:#include <stdio.h> int main() { int x, y; scanf("%d %d", &x, &y); int min = x < y ? x : y; printf("%d\n", min); return 0; }
以下是使用指针实现此功能的 C 语言程序:
```c
#include <stdio.h>
int main() {
int x, y;
scanf("%d %d", &x, &y);
int *ptr_x = &x;
int *ptr_y = &y;
int *ptr_min = (*ptr_x) < (*ptr_y) ? ptr_x : ptr_y;
printf("%d\n", *ptr_min);
return 0;
}
```
程序首先使用 `scanf` 函数从键盘输入两个整数 `x` 和 `y`,然后定义两个指针变量 `ptr_x` 和 `ptr_y`,并将它们分别指向变量 `x` 和 `y`。
接着,定义一个指针变量 `ptr_min`,使用条件运算符 `?:` 比较指针所指向的值的大小,并将较小的值所在的指针赋值给 `ptr_min`。
最后,使用 `printf` 函数输出指针变量 `ptr_min` 所指向的值,并在末尾加上换行符 `\n`。
注意,在比较指针所指向的值的大小时,需要使用 `*` 运算符取得指针所指向的值,即 `(*ptr_x) < (*ptr_y)`。
当输入样例为 `5 8` 时,程序将输出 `5`。
阅读全文
相关推荐



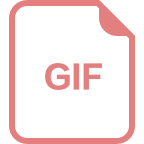

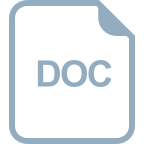











