帮我用C++写一份较为完整的公司雇佣管理系统,复杂一点,能在控制台进行操作,需要设计基类,派生类。
时间: 2024-03-04 17:48:40 浏览: 133
以下是一个可能的较为完整的公司雇佣管理系统,基于控制台进行操作:
```c++
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
// 职务类
class Job {
public:
Job(const std::string& title, double salary) : title_(title), salary_(salary) {}
const std::string& GetTitle() const { return title_; }
double GetSalary() const { return salary_; }
protected:
std::string title_;
double salary_;
};
// 基类:员工类
class Employee {
public:
Employee(const std::string& name, int age, const std::string& id) : name_(name), age_(age), id_(id) {}
const std::string& GetName() const { return name_; }
int GetAge() const { return age_; }
const std::string& GetId() const { return id_; }
virtual const Job& GetJob() const = 0; // 纯虚函数,获取职务信息
virtual void PrintInfo() const = 0; // 纯虚函数,打印员工信息
protected:
std::string name_;
int age_;
std::string id_;
};
// 派生类:技术员类
class Technician : public Employee {
public:
Technician(const std::string& name, int age, const std::string& id, const Job& job)
: Employee(name, age, id), job_(job) {}
const Job& GetJob() const override { return job_; }
void PrintInfo() const override {
std::cout << "姓名:" << GetName() << "\n年龄:" << GetAge() << "\n工号:" << GetId()
<< "\n职务:" << job_.GetTitle() << "\n薪资:" << job_.GetSalary() << "\n";
}
protected:
Job job_;
};
// 派生类:经理类
class Manager : public Employee {
public:
Manager(const std::string& name, int age, const std::string& id, const Job& job, const std::string& department)
: Employee(name, age, id), job_(job), department_(department) {}
const Job& GetJob() const override { return job_; }
void PrintInfo() const override {
std::cout << "姓名:" << GetName() << "\n年龄:" << GetAge() << "\n工号:" << GetId()
<< "\n部门:" << department_ << "\n职务:" << job_.GetTitle() << "\n薪资:" << job_.GetSalary() << "\n";
}
protected:
Job job_;
std::string department_;
};
// 公司类
class Company {
public:
Company(const std::string& name, const std::string& address) : name_(name), address_(address) {}
const std::string& GetName() const { return name_; }
const std::string& GetAddress() const { return address_; }
// 添加员工
void AddEmployee(Employee* employee) { employees_.push_back(employee); }
// 获取员工数
int GetEmployeeCount() const { return employees_.size(); }
// 获取员工信息
const Employee* GetEmployee(int index) const { return employees_[index]; }
// 输出公司信息和员工信息
void PrintInfo() const {
std::cout << "公司名称:" << GetName() << "\n公司地址:" << GetAddress() << "\n\n员工信息:\n";
for (const auto& employee : employees_) {
employee->PrintInfo();
std::cout << "\n";
}
}
protected:
std::string name_;
std::string address_;
std::vector<Employee*> employees_;
};
// 主函数
int main() {
// 创建公司和职务
Company company("ABC公司", "北京市海淀区");
Job technician_job("技术员", 5000);
Job manager_job("经理", 10000);
// 添加员工
company.AddEmployee(new Technician("张三", 25, "001", technician_job));
company.AddEmployee(new Manager("李四", 35, "002", manager_job, "市场部"));
company.AddEmployee(new Technician("王五", 30, "003", technician_job));
// 输出公司信息和员工信息
company.PrintInfo();
// 按照薪资排序并输出信息
std::sort(company.employees_.begin(), company.employees_.end(),
[](Employee* a, Employee* b) { return a->GetJob().GetSalary() > b->GetJob().GetSalary(); });
std::cout << "\n按照薪资排序后的员工信息:\n";
company.PrintInfo();
return 0;
}
```
在此实现中,除了基类、派生类和公司类,还包括了主函数,通过创建公司、添加员工、输出公司信息和员工信息等操作,实现了一个较为完整的公司雇佣管理系统。此外,还包括了一个按照薪资排序的示例,以演示如何操作员工信息。这个设计可能还有改进的空间,具体取决于应用场景和需求。
阅读全文
相关推荐
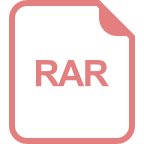
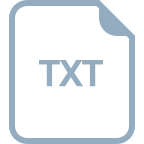

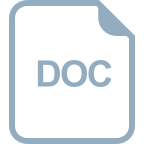
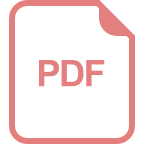
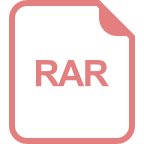
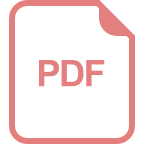
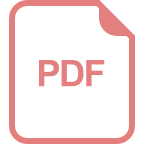
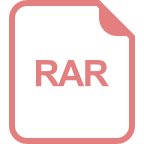
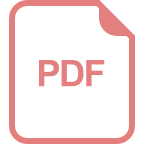
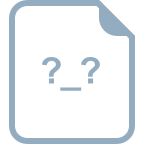
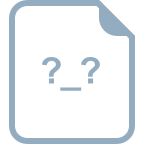
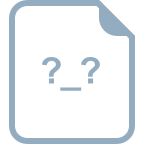
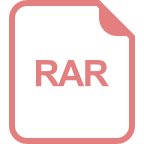
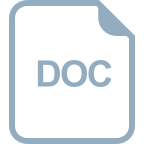
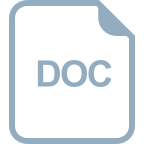
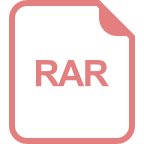