python去除字符串空格
时间: 2023-09-21 15:11:29 浏览: 38
可以使用字符串的 `strip()` 方法去除字符串两端的空格,或者使用 `rstrip()` 和 `lstrip()` 方法去除右侧和左侧的空格。例如:
```python
string_with_spaces = " hello world "
# 去除两端的空格
stripped_string = string_with_spaces.strip()
print(stripped_string) # 输出:"hello world"
# 去除右侧的空格
right_stripped_string = string_with_spaces.rstrip()
print(right_stripped_string) # 输出:" hello world"
# 去除左侧的空格
left_stripped_string = string_with_spaces.lstrip()
print(left_stripped_string) # 输出:"hello world "
```
如果想去除字符串中间的空格,可以使用 `replace()` 方法将空格替换为空。例如:
```python
string_with_spaces = "hello world"
no_spaces_string = string_with_spaces.replace(" ", "")
print(no_spaces_string) # 输出:"helloworld"
```
相关问题
python 去除字符串空格
可以使用字符串的 `strip()` 方法去除字符串开头和结尾的空格,也可以使用 `replace()` 方法去除字符串中所有的空格。示例如下:
```python
# 使用 strip() 方法去除开头和结尾的空格
s = ' hello world '
s = s.strip()
print(s) # 输出:'hello world'
# 使用 replace() 方法去除所有空格
s = ' hello world '
s = s.replace(' ', '')
print(s) # 输出:'helloworld'
```
需要注意的是,`strip()` 和 `replace()` 方法都返回新的字符串,原字符串并没有被修改。如果要修改原字符串,需要将修改后的字符串赋值给原字符串。
python 去掉字符串空格
可以使用字符串的 `strip()` 方法去掉字符串两端的空格,例如:
```python
s = " hello world "
s = s.strip()
print(s) # 输出 "hello world"
```
如果只想去掉左边或右边的空格,可以使用 `lstrip()` 或 `rstrip()` 方法。例如:
```python
s = " hello world "
s = s.lstrip()
print(s) # 输出 "hello world "
s = " hello world "
s = s.rstrip()
print(s) # 输出 " hello world"
```
相关推荐
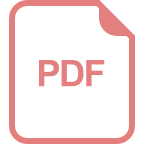
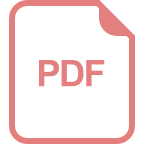
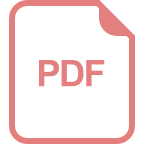








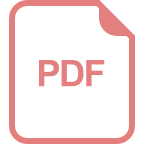