COSTAS环提取GMSK载波的python代码
时间: 2023-09-02 12:08:58 浏览: 302
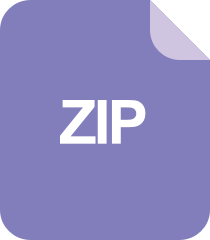
通信载波恢复中Costas环仿真的Matlab编程
以下是一个基于Python的实现COSTAS环提取MSK载波的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成GMSK调制信号
fm = 100 # 调制频率
fc = 1000 # 载波频率
BT = 0.3 # 带限时间
fs = 10 * fc # 采样频率
Ts = 1 / fs # 采样周期
Tb = 1 / fm # 一个比特的时间
N = 200 # 比特数
t = np.arange(0, N * Tb, Ts) # 时间序列
bits = np.random.randint(0, 2, N) # 生成随机的比特序列
bits[bits == 0] = -1 # 将0转换为-1
phi = np.cumsum(2 * np.pi * fc * Ts * np.ones_like(bits)) # 载波相位
s = np.zeros_like(phi)
for i in range(len(bits)):
s[i] = np.sin(phi[i]) if bits[i] == 1 else -np.sin(phi[i])
phi[i+1:] += 2 * np.pi * fc * Ts * bits[i]
h = np.exp(-np.pi * (t / TB) ** 2) / (1j * fc * Tb)
r = np.convolve(s, h, mode='same') # 加上带限滤波器
# COSTAS环提取载波相位
f0 = fc / (2 * np.pi * BT) # 等效带宽
Kp = 2 * np.pi * f0 / 100 # 相位增益
Kv = 2 * np.pi * f0 / 10 # 频率增益
theta = np.zeros_like(phi)
theta[0] = np.random.rand() * 2 * np.pi
for i in range(1, len(phi)):
error = np.imag(np.exp(-1j * theta[i-1]) * r[i])
theta[i] = theta[i-1] + Kv * error - Kp * np.sin(theta[i-1])
# 绘制原始信号和解调信号
plt.subplot(211)
plt.plot(t, s)
plt.xlabel('时间')
plt.ylabel('幅度')
plt.title('原始信号')
plt.subplot(212)
plt.plot(t, np.real(np.exp(-1j * theta) * r))
plt.xlabel('时间')
plt.ylabel('幅度')
plt.title('解调信号')
plt.tight_layout()
plt.show()
```
在上述代码中,我们首先生成了一个GMSK调制信号,然后加上了一个带限滤波器,最后使用COSTAS环提取载波相位,得到了解调信号。
在绘制图像时,我们可以看到原始信号和解调信号的波形。
阅读全文
相关推荐
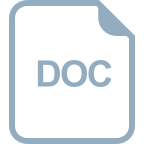
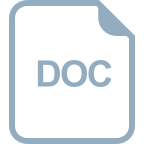

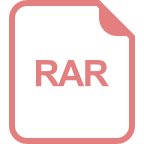
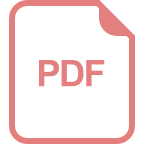
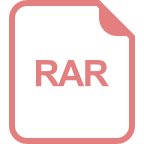
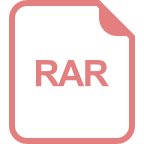
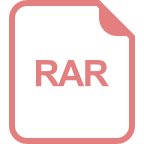






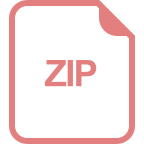
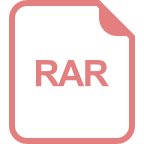
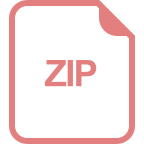
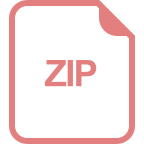