java代码xml中mysql的批量新增怎么写
时间: 2024-10-16 17:03:34 浏览: 12
在Java中,如果你想要通过XML配置文件操作MySQL数据库并实现数据的批量插入,通常会涉及到解析XML、连接数据库、读取XML元素以及执行SQL插入语句。这里是一个简单的示例,假设你有一个XML文档,其中包含一组待插入的数据:
```java
import org.w3c.dom.Document;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
import javax.xml.parsers.DocumentBuilder;
import java.sql.Connection;
import java.sql.PreparedStatement;
public void batchInsertFromXml(String xmlFilePath) {
try {
// 解析XML
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
InputSource is = new InputSource(new File(xmlFilePath));
Document doc = dBuilder.parse(is);
// 获取根节点和需要插入数据的节点列表
NodeList dataList = doc.getElementsByTagName("data");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
String sql = "INSERT INTO my_table (column1, column2, ...) VALUES (?, ?, ...)";
PreparedStatement pstmt = conn.prepareStatement(sql);
for (int i = 0; i < dataList.getLength(); i++) {
Node itemNode = dataList.item(i);
String value1 = itemNode.getTextContent().trim(); // 提取XML中的值
String value2 = ...; // 根据实际情况提取其他值
// 设置预处理语句参数
pstmt.setString(1, value1);
pstmt.setString(2, value2);
// 更多参数...
pstmt.addBatch();
}
// 执行批量插入
pstmt.executeBatch();
// 关闭资源
pstmt.close();
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
```
在这个例子中,你需要替换`xmlFilePath`为你的XML文件路径,`mydatabase`, `username`, 和 `password`为你的MySQL数据库信息,`my_table` 和列名则对应你的表结构。
阅读全文
相关推荐
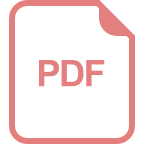
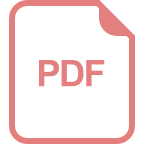
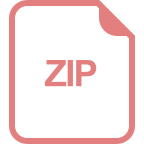
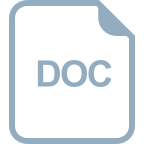
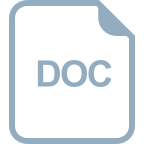
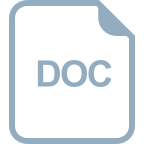
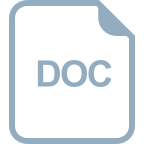




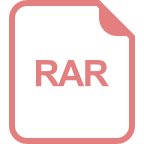
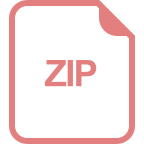
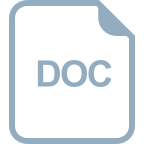
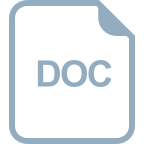



