基于python的哲学家进餐模拟程序设计
时间: 2023-07-07 18:26:36 浏览: 104
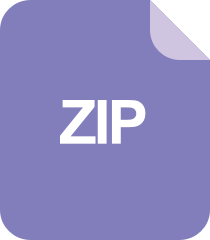
Python语言程序设计习题答案.zip

好的,下面是基于 Python 的哲学家进餐模拟程序设计。
```python
import threading
import time
class Philosopher(threading.Thread):
def __init__(self, philosopher_id, left_fork, right_fork):
threading.Thread.__init__(self)
self.philosopher_id = philosopher_id
self.left_fork = left_fork
self.right_fork = right_fork
def run(self):
while True:
self.left_fork.pick_up()
time.sleep(1)
if not self.right_fork.pick_up():
self.left_fork.put_down()
time.sleep(1)
continue
print("Philosopher ", self.philosopher_id, " is eating.")
time.sleep(1)
self.right_fork.put_down()
self.left_fork.put_down()
print("Philosopher ", self.philosopher_id, " is thinking.")
time.sleep(1)
if __name__ == '__main__':
n_philosophers = 5
forks = [threading.Lock() for _ in range(n_philosophers)]
philosophers = [Philosopher(i, forks[i], forks[(i+1)%n_philosophers]) for i in range(n_philosophers)]
for philosopher in philosophers:
philosopher.start()
```
这个程序中,我们首先定义了 `Philosopher` 类,它继承自 `threading.Thread` 类,每个哲学家通过 `left_fork` 和 `right_fork` 两个锁来控制自己的左右两个 fork。在 `run` 方法中,每个哲学家都会不断地尝试拿起左边的 fork,如果左边的 fork 没有被拿走,就等待一段时间,然后再次尝试;如果左边的 fork 被拿走了,就接着尝试拿起右边的 fork,如果右边的 fork 没有被拿走,就先放下左边的 fork,等待一段时间后再次尝试;如果右边的 fork 被拿走了,就开始进餐,并输出哲学家的 ID。在进餐完成后,哲学家会先放下右边的 fork,再放下左边的 fork,然后开始思考。
在主程序中,我们首先创建了 `n_philosophers` 个锁对象 `forks`,每个哲学家的左边的 fork 为 `forks[i]`,右边的 fork 为 `forks[(i+1)%n_philosophers]`。然后,我们创建了 `n_philosophers` 个 `Philosopher` 对象,并将它们全部启动。
这个程序中,我们使用了 `time.sleep` 方法来模拟哲学家的行为,让它们在某些情况下等待一段时间再继续执行。同时,我们也使用了 `threading.Lock` 类来实现锁的功能,避免哲学家之间互相干扰。
阅读全文
相关推荐
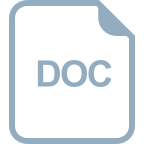
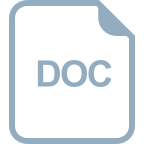
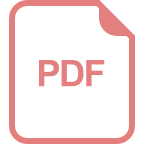
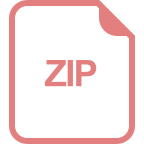
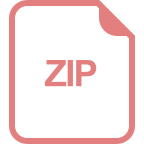
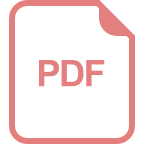
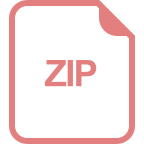
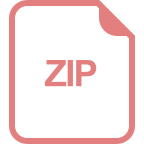
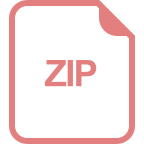
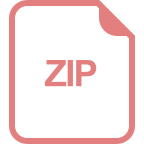
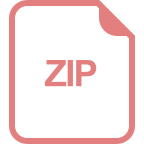
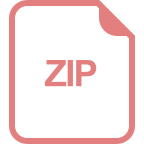
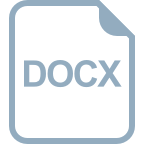
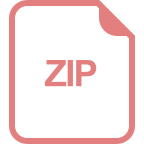