C++用栈实现幂函数并要求从文件中输入输出
时间: 2024-05-03 21:23:07 浏览: 85
这里是一个使用栈实现幂函数的示例代码,并且从文件中输入输出结果:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 100
typedef struct {
int top;
double data[STACK_SIZE];
} Stack;
void push(Stack *s, double value) {
if (s->top == STACK_SIZE) {
printf("Stack is full!\n");
exit(EXIT_FAILURE);
}
s->data[s->top++] = value;
}
double pop(Stack *s) {
if (s->top == 0) {
printf("Stack is empty!\n");
exit(EXIT_FAILURE);
}
return s->data[--s->top];
}
double power(double x, int n) {
Stack s;
s.top = 0;
if (n == 0) {
return 1.0;
}
push(&s, x);
while (n > 1) {
double value = pop(&s);
push(&s, value * value);
if (n % 2 == 1) {
push(&s, value * x);
}
n /= 2;
}
return pop(&s);
}
int main() {
FILE *fp_in = fopen("input.txt", "r");
FILE *fp_out = fopen("output.txt", "w");
if (fp_in == NULL || fp_out == NULL) {
printf("Failed to open file.\n");
exit(EXIT_FAILURE);
}
int n;
double x;
while (fscanf(fp_in, "%lf %d", &x, &n) == 2) {
double result = power(x, n);
fprintf(fp_out, "%.2lf\n", result);
}
fclose(fp_in);
fclose(fp_out);
return 0;
}
```
在这个示例代码中,我们定义了一个 `Stack` 结构体来表示栈,其中 `top` 表示栈顶的位置,`data` 数组用来存储栈中的数据。`push` 函数用于将数据压入栈中,`pop` 函数用于从栈中弹出数据。
`power` 函数使用栈来实现幂函数,首先将底数 x 压入栈中,然后不断将栈顶元素平方,直到指数 n 变为 1。在平方的过程中,如果指数 n 是奇数,就将底数 x 再次压入栈中。
在 `main` 函数中,我们打开输入和输出文件,然后循环读取输入文件中的数据,调用 `power` 函数计算幂函数的结果,并将结果写入输出文件中。最后,我们关闭文件并退出程序。
需要注意的是,在读写文件时,我们使用了 `fscanf` 和 `fprintf` 函数,它们与 `scanf` 和 `printf` 函数类似,但是可以指定文件句柄进行读写。同时,我们还需要在程序中检查文件是否成功打开,如果打开失败,就需要退出程序。
阅读全文
相关推荐

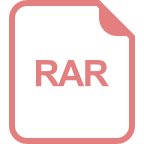
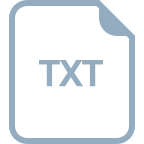
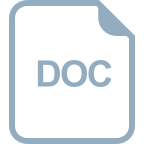
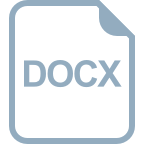
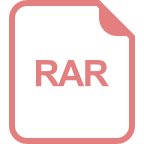
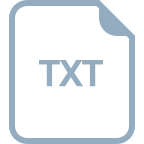
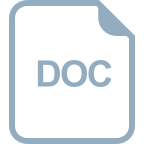
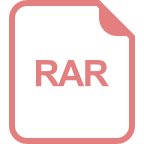
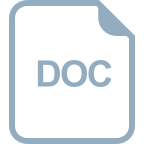
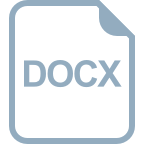
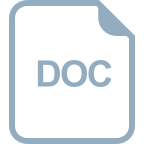
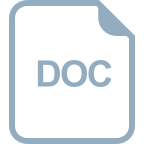
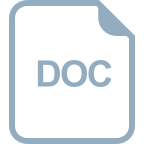
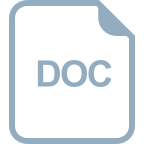