java 我现在有个list 集合,list数据里面有个时间戳字段 , 24小时范围补齐list 480条数据,代码怎么实现
时间: 2023-11-08 21:05:46 浏览: 34
你可以按照以下代码实现:
```
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// 假设 list 中的元素已经按照时间戳从小到大排序
List<Data> list = new ArrayList<>();
// ... 添加元素到 list 中
// 获取当前时间的时间戳
long currentTimestamp = System.currentTimeMillis() / 1000;
// 计算当前时间距离当天0点的时间差
long timeDiff = currentTimestamp % 86400;
// 计算当前时间距离明天0点的时间差
long nextTimeDiff = 86400 - timeDiff;
// 计算时间段的长度,这里假设为 30 分钟
int interval = 1800;
// 计算总共需要补齐的元素数量
int total = 480;
// 计算时间段的数量
int segments = 24 * 60 / (interval / 60);
// 补齐每个时间段
for (int i = 0; i < segments; i++) {
// 计算当前时间段的起始时间戳和结束时间戳
long start = currentTimestamp - timeDiff + i * interval;
long end = start + interval;
// 筛选出 list 中在当前时间段内的元素
List<Data> currentSegment = new ArrayList<>();
for (Data data : list) {
if (data.timestamp >= start && data.timestamp < end) {
currentSegment.add(data);
}
}
// 如果当前时间段内的元素数量不足 20 条,那么进行补齐
int count = currentSegment.size();
if (count < 20) {
// 计算补齐的元素数量
int missing = 20 - count;
// 使用线性插值方式生成缺失的元素
// 这里假设元素的 value 为时间戳
long step = interval / (missing + 1);
for (int j = 0; j < missing; j++) {
long timestamp = start + (j + 1) * step;
Data data = new Data();
data.timestamp = timestamp;
data.value = timestamp;
currentSegment.add(data);
}
}
// 将当前时间段的元素加入到新的 list 中
list.addAll(currentSegment);
}
// 对新的 list 按照时间戳从小到大排序
list.sort((a, b) -> Long.compare(a.timestamp, b.timestamp));
// 取出最后的 480 条元素,即为补齐后的结果
List<Data> result = list.subList(list.size() - 480, list.size());
}
static class Data {
long timestamp;
long value;
}
}
```
需要注意的是,这里使用了线性插值的方式生成缺失的元素,如果你需要采用其他方式,可以在步骤 6 中修改相应的代码即可。
相关推荐
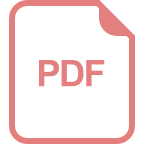














