can you write a python program to compare the difference between 2 yaml files, say, 1.yaml and 2.yaml using dictionary? The requirement is that if these 2 yaml files are identical, print the result on terminal: These 2 yaml files are identical, if these 2 yaml files are not identical, print out the difference between these 2 yaml files on termianl, thanks a lot.
时间: 2024-03-27 19:36:20 浏览: 13
Sure, I can help you with that! Here's a Python program that compares two YAML files using dictionaries and prints the result on the terminal:
```python
import yaml
def compare_yaml_files(file1, file2):
with open(file1, 'r') as f1, open(file2, 'r') as f2:
dict1 = yaml.safe_load(f1)
dict2 = yaml.safe_load(f2)
if dict1 == dict2:
print("These 2 yaml files are identical")
else:
print("These 2 yaml files are not identical")
print("Difference between these 2 yaml files:")
for key in dict1.keys() | dict2.keys():
if dict1.get(key) != dict2.get(key):
print(key + ': ' + str(dict1.get(key)) + ' != ' + str(dict2.get(key)))
file1 = '1.yaml'
file2 = '2.yaml'
compare_yaml_files(file1, file2)
```
In this program, we first open the two YAML files using the `open` function and load their contents into two dictionaries using the `yaml.safe_load` function. We then compare the two dictionaries using the `==` operator. If they are identical, we print a message indicating that. Otherwise, we iterate over the keys of both dictionaries and print out the differences between their values.
Note that this program assumes that the YAML files have the same structure and keys. If this is not the case, you may need to modify the program to handle these differences appropriately.
相关推荐
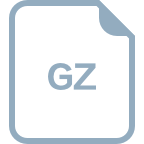















