pthread_exit如何使用
时间: 2023-06-15 16:06:20 浏览: 51
pthread_exit函数是POSIX线程库的一个函数,用于退出当前线程并返回一个值。
其使用方法如下:
```c
#include <pthread.h>
void pthread_exit(void *retval);
```
其中,参数`retval`是一个指向线程返回值的指针,它表示当前线程的返回值。可以通过这个返回值来向其他线程传递信息。
示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void* thread_function(void* arg)
{
int* value = (int*)arg;
printf("Thread function started with argument %d\n", *value);
// 设置线程返回值为100
int* ret_value = (int*)malloc(sizeof(int));
*ret_value = 100;
pthread_exit((void*)ret_value);
}
int main()
{
pthread_t thread;
int value = 42;
printf("Main program started\n");
// 创建线程并传入参数value
pthread_create(&thread, NULL, thread_function, (void*)&value);
// 等待线程结束并获取返回值
int* ret_value;
pthread_join(thread, (void**)&ret_value);
printf("Thread function returned %d\n", *ret_value);
free(ret_value);
printf("Main program exiting\n");
return 0;
}
```
在这个例子中,我们创建了一个线程,通过传入参数value来向线程传递信息。在线程函数中,我们设置了线程返回值为100,并使用pthread_exit函数来退出线程。在主函数中,我们使用pthread_join函数来等待线程结束并获取返回值,然后输出这个返回值。最后释放动态分配的内存并退出程序。
相关推荐
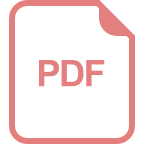














