C#引用system.xml,怎么删除节点的属性,举个例子
时间: 2023-10-19 19:05:40 浏览: 55
可以使用`XmlNode.Attributes.Remove()`方法来删除节点的属性,下面是一个示例代码:
```csharp
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load("example.xml");
XmlNode node = xmlDoc.SelectSingleNode("//node[@attribute='value']");
node.Attributes.Remove(node.Attributes["attribute"]);
xmlDoc.Save("example.xml");
```
这段代码从一个XML文件中选取一个节点,然后删除该节点的一个名为"attribute"的属性,最后保存修改后的XML文件。
相关问题
C#引用system.xml,怎么遍历一个节点的属性,然后删除其中一个属性,举个例子
假设你有以下的XML字符串:
```xml
<books>
<book id="1" author="John Doe" title="The Book" />
<book id="2" author="Jane Smith" title="Another Book" />
</books>
```
你可以使用C#的`XmlDocument`类来操作XML文件,具体操作如下:
```csharp
using System.Xml;
// 解析XML字符串
XmlDocument doc = new XmlDocument();
doc.LoadXml(xmlString);
// 获取所有book节点
XmlNodeList bookNodes = doc.GetElementsByTagName("book");
// 遍历每个book节点
foreach (XmlNode bookNode in bookNodes)
{
// 获取当前节点的id属性
XmlAttribute idAttribute = bookNode.Attributes["id"];
// 如果id属性的值为1,则删除该属性
if (idAttribute.Value == "1")
{
bookNode.Attributes.Remove(idAttribute);
}
}
// 输出修改后的XML字符串
string modifiedXmlString = doc.OuterXml;
Console.WriteLine(modifiedXmlString);
```
在上面的代码中,我们首先使用`XmlDocument`类解析XML字符串,然后获取所有的`book`节点。接着,我们遍历每个`book`节点,并获取其`id`属性。如果`id`属性的值为1,则删除该属性。最后,输出修改后的XML字符串。
c# 遍历xml所有节点和属性
当遍历XML节点时,你可以使用C#中的XmlDocument类和XmlNode类来访问节点和属性。以下是一个示例代码,演示如何遍历XML的所有节点和属性:
```csharp
using System;
using System.Xml;
class Program
{
static void Main(string[] args)
{
// 加载XML文件
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load("path_to_xml_file.xml");
// 获取XML根节点
XmlNode root = xmlDoc.DocumentElement;
// 递归遍历节点和属性
TraverseNodes(root);
Console.ReadLine();
}
static void TraverseNodes(XmlNode node)
{
// 处理当前节点
Console.WriteLine("节点名称: " + node.Name);
// 遍历属性
if (node.Attributes != null)
{
foreach (XmlAttribute attribute in node.Attributes)
{
Console.WriteLine("属性名称: " + attribute.Name + ", 属性值: " + attribute.Value);
}
}
// 遍历子节点
foreach (XmlNode childNode in node.ChildNodes)
{
TraverseNodes(childNode);
}
}
}
```
在上述代码中,我们定义了一个递归的方法`TraverseNodes`来遍历XML节点和属性。首先,我们打印当前节点的名称,然后遍历该节点的属性并打印属性的名称和值。接下来,我们使用同样的递归方法`TraverseNodes`遍历当前节点的子节点。记得将"path_to_xml_file.xml"替换为你实际的XML文件路径。你可以根据需求进行进一步处理或修改输出结果。
相关推荐
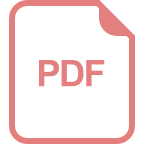
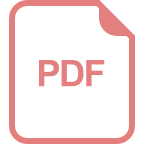
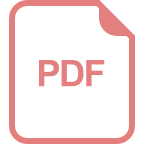












