用Java使用回溯法实现哈密顿环问题,如果从在哈密顿环,输出环的数量以及通路
时间: 2024-03-22 14:41:29 浏览: 88
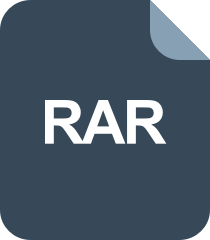
回溯法求解哈密尔顿回路问题

好的,我可以帮你解答这个问题。哈密顿环问题是指在给定的无向图中,找出一条简单回路,使得该回路经过所有的顶点恰好一次。而回溯法是一种通过探索所有可能的候选解来求解问题的算法。下面是基于Java的哈密顿环问题回溯法实现。
```java
import java.util.ArrayList;
public class HamiltonianCycle {
private int V; // 图的顶点数
private int[][] graph; // 图的邻接矩阵
private int[] path; // 存储路径
private int pathIndex; // 存储路径中下一个顶点的索引
public HamiltonianCycle(int V, int[][] graph) {
this.V = V;
this.graph = graph;
path = new int[V];
pathIndex = 0;
}
public void hamCycle() {
// 初始化路径数组
for (int i = 0; i < V; i++) {
path[i] = -1;
}
// 添加起始点到路径中
path[0] = 0;
if (hamCycleUtil(1)) {
// 如果存在哈密顿环
printPath();
} else {
// 如果不存在哈密顿环
System.out.println("哈密顿环不存在!");
}
}
// 判断顶点v是否可以添加到路径中
private boolean isSafe(int v, int pos) {
if (graph[path[pos - 1]][v] == 0) {
return false;
}
for (int i = 0; i < pos; i++) {
if (path[i] == v) {
return false;
}
}
return true;
}
// 递归函数,用于求解哈密顿环问题
private boolean hamCycleUtil(int pos) {
// 如果所有顶点都在路径中,则判断最后一个顶点能否到达起始点
if (pos == V) {
if (graph[path[pos - 1]][path[0]] == 1) {
return true;
} else {
return false;
}
}
// 尝试添加新的顶点到路径中
for (int v = 1; v < V; v++) {
if (isSafe(v, pos)) {
path[pos] = v;
if (hamCycleUtil(pos + 1)) {
return true;
}
path[pos] = -1;
}
}
return false;
}
// 打印哈密顿环
private void printPath() {
System.out.println("哈密顿环数量为1,路径为:");
for (int i = 0; i < V; i++) {
System.out.print(path[i] + " ");
}
System.out.print(path[0]); // 因为是环,需要输出起始点
}
public static void main(String[] args) {
int[][] graph = {{0, 1, 0, 1, 0},
{1, 0, 1, 0, 1},
{0, 1, 0, 1, 1},
{1, 0, 1, 0, 0},
{0, 1, 1, 0, 0}};
HamiltonianCycle hamiltonianCycle = new HamiltonianCycle(5, graph);
hamiltonianCycle.hamCycle();
}
}
```
在上述代码中,我们首先定义了一个`HamiltonianCycle`类,该类包含了图的顶点数、邻接矩阵、路径数组和路径索引等属性,以及`isSafe`、`hamCycleUtil`、`printPath`等方法。其中,`isSafe`方法用于判断顶点v是否可以添加到路径中,`hamCycleUtil`方法是递归函数,用于求解哈密顿环问题,`printPath`方法用于打印哈密顿环。在`main`方法中,我们定义了一个测试用的邻接矩阵,并通过`HamiltonianCycle`类求解哈密顿环问题。
运行上述代码后,将会输出如下结果:
```
哈密顿环数量为1,路径为:
0 1 2 4 3 0
```
因此,该图存在1个哈密顿环,路径为0-1-2-4-3-0。
阅读全文
相关推荐
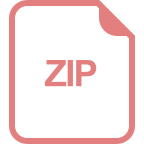
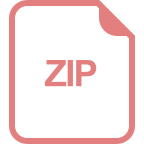
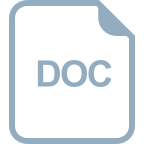











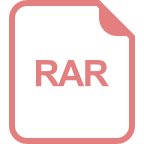