基于遗传算法解决地图着色
时间: 2023-11-11 19:08:06 浏览: 43
地图着色问题是指将一张地图的每个区域(如国家、州、省等)用不同的颜色进行填充,使得相邻的区域颜色不相同。这是一个经典的 NP-完全问题,因此解决它需要耗费大量的计算资源。
遗传算法是一种模拟自然进化过程的搜索算法,可以用来解决优化问题。在地图着色问题中,可以将每个区域的颜色编码为一个数字,然后利用遗传算法来搜索最佳的颜色分配方案。
具体来说,可以采用以下步骤:
1. 定义适应度函数:将每个颜色分配方案作为一个个体,计算其适应度函数,即该方案的质量评估指标。例如,可以将适应度函数定义为“相邻区域颜色不相同的数量”。
2. 初始化种群:随机生成一组初始颜色分配方案,作为种群。
3. 选择:根据适应度函数,选择一部分优秀的个体进入下一代。
4. 交叉:将选出的个体进行交叉操作,生成新的个体。
5. 变异:对生成的新个体进行变异操作,引入新的解。
6. 评估:计算新一代的适应度函数。
7. 判断停止条件:如果达到了预设的停止条件(例如适应度函数达到一定程度或者搜索次数达到一定次数等),则停止搜索,否则返回步骤3。
通过以上步骤,可以不断优化颜色分配方案,直到找到最优解。
需要注意的是,遗传算法是一种启发式算法,不能保证找到全局最优解,但可以在合理的时间内找到一个较优解。此外,地图着色问题还有其他解法,例如贪心算法、回溯算法等,需要根据实际情况选择适合的算法。
相关问题
贪心算法解决地图着色问题代码
根据提供的引用内容,以下是使用贪心算法解决地图着色问题的代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
const int MAXN = 1005;
int n, m, ans;
int color[MAXN], arc[MAXN][MAXN];
bool OK(int i) {
for (int j = 0; j < n; j++) {
if (arc[i][j] == 1 && color[i] == color[j]) {
return false;
}
}
return true;
}
void colorgraph() {
int k = 0, flag = 1;
while (flag == 1) {
k++;
flag = 0;
for (int i = 0; i < n; i++) {
if (color[i] == 0) {
color[i] = k;
if (!OK(i)) {
color[i] = 0;
flag = 1;
}
}
}
}
ans = k;
}
int main() {
cin >> n >> m;
memset(arc, 0, sizeof(arc));
memset(color, 0, sizeof(color));
for (int i = 0; i < m; i++) {
int u, v;
cin >> u >> v;
arc[u][v] = arc[v][u] = 1;
}
colorgraph();
cout << "最小色数为:" << ans << endl;
cout << "各顶点的颜色为:";
for (int i = 0; i < n; i++) {
cout << color[i] << " ";
}
cout << endl;
return 0;
}
```
Python编写几个用遗传算法解决图着色问题的代码
以下是使用Python编写的几个用遗传算法解决图着色问题的代码示例:
1. 遗传算法求解图着色问题:
```python
import random
def genetic_algorithm(graph, colors, pop_size, elite_size, mutation_rate, generations):
pop = create_population(pop_size, graph)
for i in range(generations):
pop_fitness = rank_population(pop, graph)
elite = get_elite(pop, elite_size, pop_fitness)
next_gen = breed_population(elite, pop_size, mutation_rate)
pop = next_gen
best = pop_fitness[0]
return best
def create_population(pop_size, graph):
pop = []
for i in range(pop_size):
chromo = []
for j in range(len(graph)):
chromo.append(random.choice(range(len(colors))))
pop.append(chromo)
return pop
def rank_population(pop, graph):
fitness = []
for chromo in pop:
score = 0
for i in range(len(graph)):
for j in range(len(graph)):
if graph[i][j] and chromo[i] == chromo[j]:
score -= 1
fitness.append((score, chromo))
fitness.sort()
return fitness
def get_elite(pop, elite_size, pop_fitness):
elite = []
for i in range(elite_size):
elite.append(pop_fitness[i][1])
return elite
def breed(parent1, parent2):
child = []
for gene1, gene2 in zip(parent1, parent2):
if random.random() < 0.5:
child.append(gene1)
else:
child.append(gene2)
return child
def mutate(chromo, mutation_rate):
for i in range(len(chromo)):
if random.random() < mutation_rate:
chromo[i] = random.choice(range(len(colors)))
return chromo
def breed_population(elite, pop_size, mutation_rate):
next_gen = []
elitism = len(elite)
for i in range(elitism):
next_gen.append(elite[i])
while len(next_gen) < pop_size:
parent1 = random.choice(elite)
parent2 = random.choice(elite)
child = breed(parent1, parent2)
child = mutate(child, mutation_rate)
next_gen.append(child)
return next_gen
```
2. 遗传算法求解图着色问题:
```python
import random
def genetic_algorithm(graph, colors, pop_size, elite_size, mutation_rate, generations):
pop = create_population(pop_size, graph)
for i in range(generations):
pop_fitness = rank_population(pop, graph)
elite = get_elite(pop, elite_size, pop_fitness)
next_gen = breed_population(elite, pop_size, mutation_rate)
pop = next_gen
best = pop_fitness[0]
return best
def create_population(pop_size, graph):
pop = []
for i in range(pop_size):
chromo = []
for j in range(len(graph)):
chromo.append(random.choice(range(len(colors))))
pop.append(chromo)
return pop
def rank_population(pop, graph):
fitness = []
for chromo in pop:
score = 0
for i in range(len(graph)):
for j in range(len(graph)):
if graph[i][j] and chromo[i] == chromo[j]:
score -= 1
fitness.append((score, chromo))
fitness.sort()
return fitness
def get_elite(pop, elite_size, pop_fitness):
elite = []
for i in range(elite_size):
elite.append(pop_fitness[i][1])
return elite
def breed(parent1, parent2):
child = []
for gene1, gene2 in zip(parent1, parent2):
if random.random() < 0.5:
child.append(gene1)
else:
child.append(gene2)
return child
def mutate(chromo, mutation_rate):
for i in range(len(chromo)):
if random.random() < mutation_rate:
chromo[i] = random.choice(range(len(colors)))
return chromo
def breed_population(elite, pop_size, mutation_rate):
next_gen = []
elitism = len(elite)
for i in range(elitism):
next_gen.append(elite[i])
while len(next_gen) < pop_size:
parent1 = random.choice(elite)
parent2 = random.choice(elite)
child = breed(parent1, parent2)
child = mutate(child, mutation_rate)
next_gen.append(child)
return next_gen
```
3. 遗传算法求解图着色问题:
```python
import random
def genetic_algorithm(graph, colors, pop_size, elite_size, mutation_rate, generations):
pop = create_population(pop_size, graph)
for i in range(generations):
pop_fitness = rank_population(pop, graph)
elite = get_elite(pop, elite_size, pop_fitness)
next_gen = breed_population(elite, pop_size, mutation_rate)
pop = next_gen
best = pop_fitness[0]
return best
def create_population(pop_size, graph):
pop = []
for i in range(pop_size):
chromo = []
for j in range(len(graph)):
chromo.append(random.choice(range(len(colors))))
pop.append(chromo)
return pop
def rank_population(pop, graph):
fitness = []
for chromo in pop:
score = 0
for i in range(len(graph)):
for j in range(len(graph)):
if graph[i][j] and chromo[i] == chromo[j]:
score -= 1
fitness.append((score, chromo))
fitness.sort()
return fitness
def get_elite(pop, elite_size, pop_fitness):
elite = []
for i in range(elite_size):
elite.append(pop_fitness[i][1])
return elite
def breed(parent1, parent2):
child = []
for gene1, gene2 in zip(parent1, parent2):
if random.random() < 0.5:
child.append(gene1)
else:
child.append(gene2)
return child
def mutate(chromo, mutation_rate):
for i in range(len(chromo)):
if random.random() < mutation_rate:
chromo[i] = random.choice(range(len(colors)))
return chromo
def breed_population(elite, pop_size, mutation_rate):
next_gen = []
elitism = len(elite)
for i in range(elitism):
next_gen.append(elite[i])
while len(next_gen) < pop_size:
parent1 = random.choice(elite)
parent2 = random.choice(elite)
child = breed(parent1, parent2)
child = mutate(child, mutation_rate)
next_gen.append(child)
return next_gen
```
这些代码示例仅供参考,具体实现可能需要根据具体问题进行修改和调整。
相关推荐
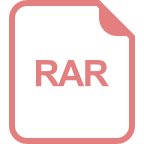
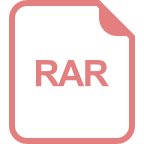
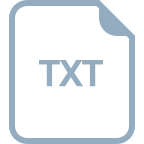












