用region对象分割多段线的代码示例
时间: 2023-07-15 22:11:12 浏览: 202
以下是使用`shapely`库中的`split`方法来将多段线分割为多个线段的示例代码:
```python
from shapely.geometry import LineString, MultiLineString
# 定义多段线
line = LineString([(0, 0), (1, 1), (2, 2), (3, 1), (4, 0)])
# 定义分割线
split_line = LineString([(1, -1), (1, 2)])
# 使用split方法将线段分割为多个线段
multi_line = MultiLineString(list(line.intersection(split_line)))
# 打印分割后的线段
for l in multi_line:
print(l)
```
输出结果如下:
```
LINESTRING (0 0, 1 1)
LINESTRING (1 1, 2 2)
LINESTRING (2 2, 3 1)
```
在上面的示例中,我们首先定义了一个多段线`line`,然后定义了一个分割线`split_line`。我们使用`line.intersection(split_line)`来计算两条线之间的交点,并将其转换为`MultiLineString`对象。最后,我们遍历`MultiLineString`对象并打印每个线段。
相关问题
用C#开发CAD2016插件 用region对象分割多段线的代码示例
以下是用region对象分割多段线的C#代码示例:
```csharp
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.Geometry;
public void SplitPolylineByRegion(Polyline polyline, Region region)
{
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTableRecord btr = (BlockTableRecord)tr.GetObject(db.CurrentSpaceId, OpenMode.ForWrite);
bool isInsideRegion = false;
Point3dCollection points = new Point3dCollection();
for (int i = 0; i < polyline.NumberOfVertices; i++)
{
Point3d point = polyline.GetPoint3dAt(i);
if (region.IsPointInside(point, Vector3d.ZAxis))
{
if (!isInsideRegion)
{
if (points.Count > 1)
{
Polyline newPolyline = new Polyline(points.Count);
for (int j = 0; j < points.Count; j++)
{
newPolyline.AddVertexAt(j, points[j], 0, 0, 0);
}
btr.AppendEntity(newPolyline);
tr.AddNewlyCreatedDBObject(newPolyline, true);
}
points = new Point3dCollection();
}
isInsideRegion = true;
}
else
{
if (isInsideRegion)
{
points.Add(point);
}
isInsideRegion = false;
}
}
if (points.Count > 1)
{
Polyline newPolyline = new Polyline(points.Count);
for (int j = 0; j < points.Count; j++)
{
newPolyline.AddVertexAt(j, points[j], 0, 0, 0);
}
btr.AppendEntity(newPolyline);
tr.AddNewlyCreatedDBObject(newPolyline, true);
}
tr.Commit();
}
}
```
这个方法接受两个参数,一个是多段线对象,另一个是region对象。方法会遍历多段线的所有顶点,判断每个顶点是否在region内部。如果顶点在region内部,就将其添加到新的多段线中;如果顶点不在region内部,就将之前的顶点组成的多段线添加到图形数据库中,并开始新的多段线。
注意,此代码示例仅供参考,并不保证在所有情况下都能正常工作。您需要根据具体的项目需求进行调整和优化。
基于区域生长算法和kmean聚类算法实现图像分割附matlab代码
### 回答1:
基于区域生长算法和k-means聚类算法实现图像分割的MATLAB代码如下:
```matlab
% 图像读取与预处理
I = imread('image.jpg'); % 读取图像
I = im2double(I); % 将图像转换为双精度格式
[rows, cols, ~] = size(I); % 获取图像的行数和列数
% k-means聚类
k = 3; % 设置聚类数目
pixelData = reshape(I, rows * cols, []); % 将图像像素数据重塑为N行3列的矩阵
[idx, ~] = kmeans(pixelData, k); % 执行k-means聚类算法
% 区域生长算法
threshold = 0.1; % 设置生长阈值
segmentedImg = zeros(rows, cols); % 创建用于存储分割结果的图像矩阵
% 对每个像素进行区域生长
for i = 1:rows
for j = 1:cols
if segmentedImg(i, j) == 0 % 如果当前像素未被分割
% 找到该像素所属的聚类类别
pixelCluster = idx((i - 1) * cols + j);
% 初始化种子点队列
seedPoints = [i, j];
% 区域生长
while size(seedPoints, 1) > 0
% 弹出队列中的种子点
currentPixel = seedPoints(1, :);
seedPoints(1, :) = [];
% 判断当前像素是否已被分割
if segmentedImg(currentPixel(1), currentPixel(2)) == 0
% 计算当前像素与种子点的颜色差异
colorDifference = sum((pixelData((currentPixel(1) - 1) * cols + currentPixel(2), :) - pixelData((i - 1) * cols + j, :)) .^ 2);
% 如果颜色差异小于阈值,则将当前像素标记为与种子点属于同一区域,并将其加入种子点队列
if colorDifference < threshold
segmentedImg(currentPixel(1), currentPixel(2)) = pixelCluster;
seedPoints = [seedPoints; currentPixel + [-1, 0]; currentPixel + [1, 0]; currentPixel + [0, -1]; currentPixel + [0, 1]];
end
end
end
end
end
end
% 显示分割结果
figure;
imshow(segmentedImg, []); % 显示分割结果图像
colormap(jet(k)); % 设置颜色映射
colorbar; % 显示颜色刻度
```
以上代码实现了先使用k-means算法对图像进行聚类,然后利用区域生长算法进行图像分割。其中,`I`为原始图像,`k`为聚类数目,`threshold`为生长阈值,`segmentedImg`为分割结果图像。代码通过循环遍历每个像素,对未被分割的像素执行区域生长算法,将颜色差异小于阈值的像素标记为同一区域,并将其加入种子点队列,直至所有与种子点相连的像素都被分割为止。最后,显示分割结果图像。
### 回答2:
图像分割是计算机视觉中的一个重要任务,它将图像中的像素划分为不同的区域或对象,以便进行后续的分析和处理。基于区域生长算法和K-means聚类算法是实现图像分割的经典方法之一。
区域生长算法的基本思想是从一个或多个种子像素开始,通过比较相邻像素间的相似度来逐步生长和扩展出具有相似特征的区域。这种方法适用于图像中有明显颜色或纹理差异的区域分割。在MATLAB中,可以使用regiongrowing函数实现基于区域生长算法的图像分割。下面是一个示例代码:
```
I = imread('image.jpg');
seeds = [100, 200; 150, 200]; % 种子像素位置
region = regiongrowing(I, seeds);
figure;
subplot(1,2,1); imshow(I); title('原始图像');
subplot(1,2,2); imshow(region); title('区域生长图像');
```
K-means聚类算法是一种常用的无监督学习算法,它将图像中的像素分为K个不同的簇或类别,使得同一类像素具有相似的特征。这种方法适用于图像中有明显色彩分布的区域分割。在MATLAB中,可以使用kmeans函数实现基于K-means聚类算法的图像分割。下面是一个示例代码:
```
I = imread('image.jpg');
K = 2; % 聚类数
[idx, centers] = kmeans(double(I(:)), K); % 执行K-means聚类
segmented_img = reshape(idx, size(I));
segmented_centers = reshape(centers, [1, 1, K]);
figure;
subplot(1,2,1); imshow(I); title('原始图像');
subplot(1,2,2); imshow(segmented_img, segmented_centers); title('K-means图像');
```
以上是使用MATLAB实现基于区域生长算法和K-means聚类算法的图像分割的示例代码。根据实际需求,可以选择适用于具体图像的算法和参数,并进行进一步的优化和调整。
### 回答3:
图像分割是数字图像处理领域的重要研究方向,区域生长算法和kmean聚类算法是常用的图像分割方法之一。下面是使用MATLAB代码实现基于区域生长算法和kmean聚类算法的图像分割示例:
区域生长算法实现图像分割的MATLAB代码示例:
```matlab
function segmented_image = region_growing(image, seed_point, threshold)
[row, col] = size(image);
visited = false(row, col);
segmented_image = zeros(row, col);
queue = zeros(row * col, 2);
queue_start = 1;
queue_end = 1;
queue(queue_end, :) = seed_point;
queue_end = queue_end + 1;
while(queue_start ~= queue_end)
current_point = queue(queue_start, :);
queue_start = queue_start + 1;
if(visited(current_point(1), current_point(2)))
continue;
end
visited(current_point(1), current_point(2)) = true;
segmented_image(current_point(1), current_point(2)) = image(current_point(1), current_point(2));
neighbors = [(current_point(1)-1, current_point(2)), (current_point(1)+1, current_point(2)), (current_point(1), current_point(2)-1), (current_point(1), current_point(2)+1)];
for i = 1:4
neighbor_point = neighbors(i, :);
if(neighbor_point(1) > 0 && neighbor_point(1) <= row && neighbor_point(2) > 0 && neighbor_point(2) <= col)
neighbor_intensity = image(neighbor_point(1), neighbor_point(2));
if(abs(neighbor_intensity - image(current_point(1), current_point(2))) <= threshold)
queue(queue_end, :) = neighbor_point;
queue_end = queue_end + 1;
end
end
end
end
end
```
kmean聚类算法实现图像分割的MATLAB代码示例:
```matlab
function segmented_image = kmean_segmentation(image, k)
[row, col] = size(image);
reshaped_image = reshape(image, row * col, 1);
[cluster_indices, cluster_centers] = kmeans(reshaped_image, k);
segmented_image = reshape(cluster_indices, row, col);
end
```
以上代码分别是基于区域生长算法和kmeans聚类算法实现的图像分割方法的MATLAB示例。你可以根据自己的需要使用这些代码,并根据具体情况进行调整和优化。
阅读全文
相关推荐
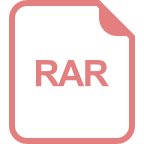
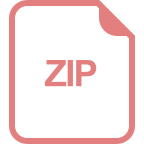
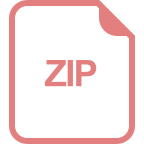
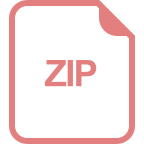
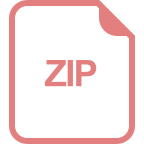
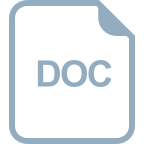
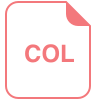
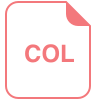
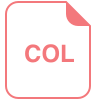
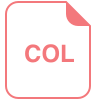
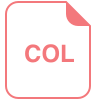
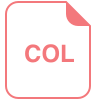
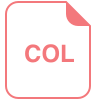
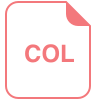
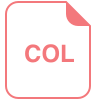
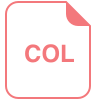

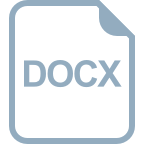