Java 根据Map的key对map进行排序
时间: 2023-05-12 21:07:16 浏览: 101
可以使用TreeMap来实现根据Map的key进行排序,TreeMap是基于红黑树实现的,可以自动按照key的自然顺序排序。以下是一个示例代码:
```java
Map<String, Integer> map = new TreeMap<>();
map.put("c", 3);
map.put("a", 1);
map.put("b", 2);
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
```
输出结果为:
```
a : 1
相关问题
Java 使用stream流根据Map的key对map进行排序
可以使用以下代码对Map按照key进行排序:
Map<String, Integer> map = new HashMap<>();
// 添加元素到map中
Map<String, Integer> sortedMap = map.entrySet()
.stream()
.sorted(Map.Entry.comparingByKey())
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue,
(oldValue, newValue) -> oldValue, LinkedHashMap::new));
java stream map key 排序
Java Stream可以方便地对数据进行处理和操作。其中map()方法用于将Stream中的每个元素映射为另一个元素,并返回一个新的Stream。通常,对于这样的操作,我们需要对结果进行排序以便更好地分析和使用。那么如何对Java Stream中的map结果进行排序呢?
在Java 8中,我们可以使用sorted()方法来排序Stream中的元素。对于Map对象,我们可以在map()方法中使用lambda表达式来实现Key排序。示例如下:
```
Map<String, Integer> map = new HashMap<>();
map.put("Apple", 10);
map.put("Orange", 20);
map.put("Banana", 5);
map.put("Pear", 15);
// Key排序
Map<String, Integer> sortedMap = map.entrySet().stream()
.sorted(Map.Entry.comparingByKey())
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue,
(oldValue, newValue) -> oldValue, LinkedHashMap::new));
System.out.println(sortedMap);
```
在上面的示例中,使用entrySet()方法将Map转化为Stream,然后使用sorted()方法对Key进行排序。最后使用collect()方法将结果存入LinkHashMap中,因为LinkHashMap可以保证插入顺序。
如果我们需要对Value进行排序,只需要将comparingByKey()替换为comparingByValue()即可。
以上就是Java Stream中对Map进行Key排序的方法。通过使用lambda表达式和Stream API,我们可以方便地进行数据处理和操作。
阅读全文
相关推荐
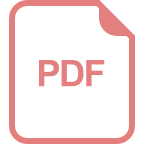
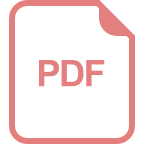
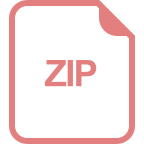
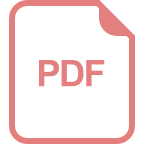













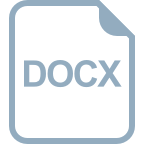