根据key对map 排序
时间: 2023-08-04 08:03:44 浏览: 100
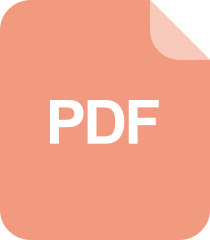
Java Map 按key排序和按Value排序的实现方法
你可以通过以下步骤按键对 Map 进行排序:
1. 将 Map 转换为 List,该 List 包含 Map 中的键值对。
2. 创建一个比较器(Comparator)来定义按键排序的规则。
3. 使用 Collections.sort() 方法并传入 List 和比较器来对列表进行排序。
4. 将排序后的列表转换回 Map。
以下是一个示例代码:
```java
import java.util.*;
public class MapSortExample {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(1, "Apple");
map.put(3, "Orange");
map.put(2, "Banana");
List<Map.Entry<Integer, String>> list = new ArrayList<>(map.entrySet());
// 使用比较器按键排序
Collections.sort(list, new Comparator<Map.Entry<Integer, String>>() {
public int compare(Map.Entry<Integer, String> o1, Map.Entry<Integer, String> o2) {
return o1.getKey().compareTo(o2.getKey());
}
});
// 将排序后的列表转换回 Map
Map<Integer, String> sortedMap = new LinkedHashMap<>();
for (Map.Entry<Integer, String> entry : list) {
sortedMap.put(entry.getKey(), entry.getValue());
}
// 打印排序后的 Map
for (Map.Entry<Integer, String> entry : sortedMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
运行以上代码,输出将会是按键排序后的 Map:
```
1: Apple
2: Banana
3: Orange
```
请注意,该示例代码使用了整数作为键,但你也可以根据需要进行调整,以适应不同类型的键。
阅读全文
相关推荐
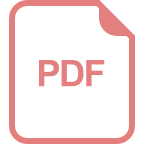

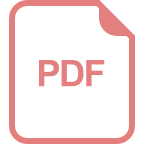













