深度神经网络——中文语音识别 代码实现
时间: 2023-09-02 11:05:46 浏览: 85
中文语音识别是一个复杂的任务,需要大量的数据和计算资源。以下是一个示例代码,用于演示如何使用深度神经网络进行中文语音识别。
首先,我们需要导入必要的库和数据集。在这里,我们使用了 THCHS-30 数据集,该数据集包含了大约 10 小时的中文语音数据。
```python
import os
import numpy as np
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout, LSTM, TimeDistributed, Activation, Bidirectional, BatchNormalization
from tensorflow.keras.callbacks import ModelCheckpoint
from tensorflow.keras.utils import to_categorical
from python_speech_features import mfcc
from tqdm import tqdm
# Load THCHS-30 dataset
def load_data():
data = []
labels = []
label_map = {}
mfcc_feature_len = 13
with open('data/thchs30/data' + os.sep + 'data' + os.sep + 'train' + os.sep + 'train.wav.lst', 'r') as f:
lines = f.readlines()
for line in tqdm(lines):
parts = line.strip().split()
wav_file = 'data/thchs30/data' + os.sep + 'train' + os.sep + parts[0] + '.wav'
label_file = 'data/thchs30/data' + os.sep + 'train' + os.sep + parts[0] + '.trn'
with open(label_file, 'r') as f2:
label = f2.read().strip()
if label not in label_map:
label_map[label] = len(label_map)
labels.append(label_map[label])
signal, rate = tf.audio.decode_wav(tf.io.read_file(wav_file))
signal = tf.squeeze(signal, axis=-1)
mfcc_features = mfcc(signal.numpy(), rate.numpy(), numcep=mfcc_feature_len)
data.append(mfcc_features)
return np.array(data), np.array(labels), label_map
# Load data
data, labels, label_map = load_data()
num_labels = len(label_map)
```
接下来,我们需要将数据集分成训练集和测试集,并将标签转换为 one-hot 编码。
```python
train_ratio = 0.8
num_train = int(len(data) * train_ratio)
# Split data into train and test sets
train_data = data[:num_train]
train_labels = labels[:num_train]
test_data = data[num_train:]
test_labels = labels[num_train:]
# Convert labels to one-hot encoding
train_labels = to_categorical(train_labels, num_labels)
test_labels = to_categorical(test_labels, num_labels)
```
然后,我们可以构建一个简单的双向 LSTM 模型。
```python
model = Sequential()
model.add(Bidirectional(LSTM(128, return_sequences=True), input_shape=(None, mfcc_feature_len)))
model.add(Dropout(0.2))
model.add(Bidirectional(LSTM(128)))
model.add(Dropout(0.2))
model.add(Dense(num_labels))
model.add(Activation('softmax'))
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
```
我们还可以添加一些回调函数,以便在训练过程中自动保存最佳模型。
```python
# Define checkpoint callback
checkpoint_callback = ModelCheckpoint('model.h5', monitor='val_accuracy', save_best_only=True, mode='max')
# Train model
history = model.fit(train_data, train_labels, batch_size=32, epochs=100, validation_data=(test_data, test_labels), callbacks=[checkpoint_callback])
```
最后,我们可以使用训练好的模型进行预测。
```python
# Load best model
model.load_weights('model.h5')
# Predict labels for test data
predicted_labels = model.predict(test_data)
# Convert predicted labels to integers
predicted_labels = np.argmax(predicted_labels, axis=-1)
# Convert labels back to text
predicted_labels = [list(label_map.keys())[list(label_map.values()).index(label)] for label in predicted_labels]
```
这就是一个简单的中文语音识别模型的实现。当然,为了达到更好的性能,我们需要更复杂的模型和更多的数据。
相关推荐
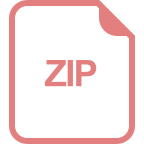
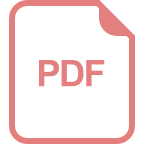
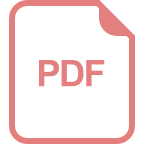













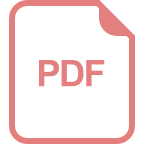
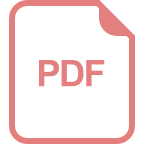