android BLE扫描、连接、切断、添加蓝牙权限的代码
时间: 2023-07-10 14:06:14 浏览: 210
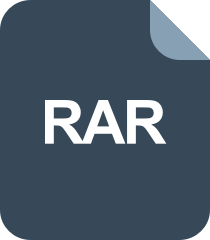
Android 蓝牙 BLE4.0代码

以下是Android BLE扫描、连接、切断、添加蓝牙权限的代码示例:
#### 添加蓝牙权限
在AndroidManifest.xml文件中添加以下蓝牙权限:
```xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.bluetooth">
<uses-feature android:name="android.hardware.bluetooth_le" android:required="true"/>
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<application
...
</application>
</manifest>
```
#### 扫描BLE设备
```java
private BluetoothAdapter bluetoothAdapter;
private BluetoothLeScanner bluetoothLeScanner;
private ScanCallback scanCallback;
// 初始化蓝牙适配器和扫描回调
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
bluetoothLeScanner = bluetoothAdapter.getBluetoothLeScanner();
scanCallback = new ScanCallback() {
@Override
public void onScanResult(int callbackType, ScanResult result) {
super.onScanResult(callbackType, result);
// 处理扫描结果
}
@Override
public void onScanFailed(int errorCode) {
super.onScanFailed(errorCode);
// 处理扫描失败
}
};
// 开始扫描BLE设备
ScanFilter scanFilter = new ScanFilter.Builder()
.setDeviceName("MyDevice")
.build();
List<ScanFilter> scanFilters = new ArrayList<>();
scanFilters.add(scanFilter);
ScanSettings scanSettings = new ScanSettings.Builder()
.setScanMode(ScanSettings.SCAN_MODE_LOW_POWER)
.build();
bluetoothLeScanner.startScan(scanFilters, scanSettings, scanCallback);
```
#### 连接BLE设备
```java
private BluetoothGatt bluetoothGatt;
// 连接BLE设备
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress);
bluetoothGatt = device.connectGatt(this, false, gattCallback);
// 处理连接回调
private final BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
super.onConnectionStateChange(gatt, status, newState);
if (newState == BluetoothProfile.STATE_CONNECTED) {
// 连接成功
bluetoothGatt.discoverServices();
} else {
// 连接失败
bluetoothGatt.close();
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
super.onServicesDiscovered(gatt, status);
// 处理服务发现结果
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
super.onCharacteristicRead(gatt, characteristic, status);
// 处理读取特征值结果
}
@Override
public void onCharacteristicWrite(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
super.onCharacteristicWrite(gatt, characteristic, status);
// 处理写入特征值结果
}
@Override
public void onCharacteristicChanged(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic) {
super.onCharacteristicChanged(gatt, characteristic);
// 处理特征值变化通知
}
};
```
#### 切断BLE设备
```java
// 断开BLE设备连接
bluetoothGatt.disconnect();
// 处理断开连接回调
private final BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
super.onConnectionStateChange(gatt, status, newState);
if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 断开连接成功
bluetoothGatt.close();
}
}
};
```
阅读全文
相关推荐
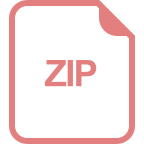
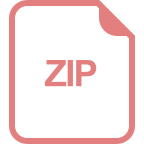
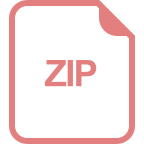
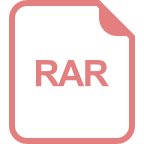
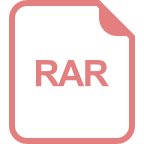
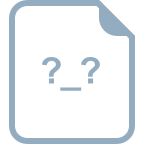
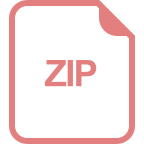
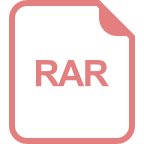
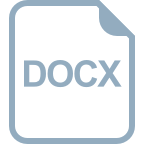
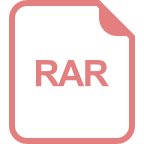
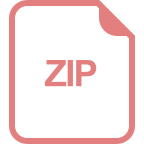
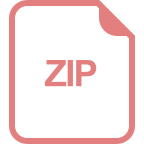
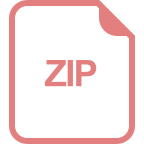
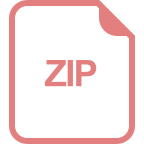
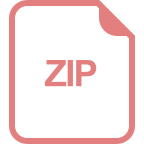
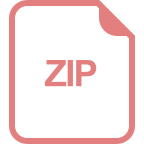
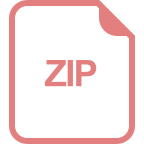