p ython isalpha
时间: 2024-01-17 15:04:45 浏览: 27
`isalpha()` 是 Python 中的一个字符串方法,用于判断一个字符串是否只由字母组成。如果字符串只由字母组成,则返回 True,否则返回 False。
例如,以下代码将返回 True:
```
string = "hello"
print(string.isalpha())
```
而以下代码将返回 False:
```
string = "hello123"
print(string.isalpha())
```
相关问题
p ython凯撒加密解密
这里提供一个简单的Python实现凯撒加密和解密的代码:
```python
def caesar_encrypt(plaintext, shift):
"""
凯撒加密函数
:param plaintext: 明文
:param shift: 密钥
:return: 密文
"""
ciphertext = ""
for char in plaintext:
if char.isalpha():
# 将字符转换为 ASCII 码
char_code = ord(char)
# 判断字符是大写字母还是小写字母
if char.isupper():
# 对大写字母进行加密
char_code = (char_code - 65 + shift) % 26 + 65
else:
# 对小写字母进行加密
char_code = (char_code - 97 + shift) % 26 + 97
# 将 ASCII 码转换回字符
char = chr(char_code)
ciphertext += char
return ciphertext
def caesar_decrypt(ciphertext, shift):
"""
凯撒解密函数
:param ciphertext: 密文
:param shift: 密钥
:return: 明文
"""
plaintext = ""
for char in ciphertext:
if char.isalpha():
# 将字符转换为 ASCII 码
char_code = ord(char)
# 判断字符是大写字母还是小写字母
if char.isupper():
# 对大写字母进行解密
char_code = (char_code - 65 - shift) % 26 + 65
else:
# 对小写字母进行解密
char_code = (char_code - 97 - shift) % 26 + 97
# 将 ASCII 码转换回字符
char = chr(char_code)
plaintext += char
return plaintext
```
使用方法:
```python
plaintext = "Hello World!"
shift = 3
ciphertext = caesar_encrypt(plaintext, shift)
print("加密后的密文:", ciphertext)
decrypted_text = caesar_decrypt(ciphertext, shift)
print("解密后的明文:", decrypted_text)
```
输出结果:
```
加密后的密文: Khoor Zruog!
解密后的明文: Hello World!
```
其中,`plaintext` 表示明文字符串,`shift` 表示密钥,`ciphertext` 表示加密后的密文字符串,`decrypted_text` 表示解密后的明文字符串。
p ython 实现凯撒加密
好的,以下是 Python 实现凯撒加密的代码:
```python
def caesar_cipher(text, shift):
result = ""
for char in text:
if char.isalpha():
ascii_code = ord(char)
shifted_ascii_code = ascii_code + shift
if char.isupper():
if shifted_ascii_code > ord('Z'):
shifted_ascii_code -= 26
elif shifted_ascii_code < ord('A'):
shifted_ascii_code += 26
else:
if shifted_ascii_code > ord('z'):
shifted_ascii_code -= 26
elif shifted_ascii_code < ord('a'):
shifted_ascii_code += 26
result += chr(shifted_ascii_code)
else:
result += char
return result
```
其中,`text` 是要加密的文本,`shift` 是加密的偏移量。函数会返回加密后的文本。
例如,我们可以这样调用函数:
```python
text = "Hello, World!"
shift = 3
encrypted_text = caesar_cipher(text, shift)
print(encrypted_text)
```
输出结果为:
```
Khoor, Zruog!
```
这就是使用凯撒加密算法加密后的文本。
相关推荐
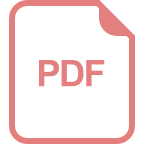
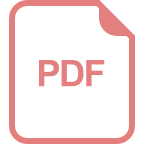
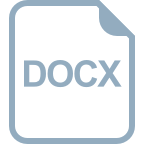












