def test(a): if 'P' not in a or 'T' not in a: # 如果字符串中没有P或者T,则返回0 return 0 x = a.index('P') # 找到P的索引 y = a.index('T') # 找到T的索引 if x > y: # P在T的后面,返回0 return 0 if y == x + 1: # P和T之间没有字符,返回0 return 0 b = a[:x] # P之前的字符串 c = a[x + 1:y] # P和T之间的字符串 d = a[y + 1:] # T之后的字符串 if not b.isalpha() or not c.isalpha() or not d.isalpha(): # b、c、d中只能包含字母A return 0 if d == b * len(c): # 条件判断 return 1 else: return 0 n = int(input()) for _ in range(n): s = input() if test(s) == 1: print('YES') else: print('NO')
时间: 2024-04-16 13:29:40 浏览: 88
这是一个判断字符串是否符合一定条件的代码。首先,它会读取一个整数 n,表示接下来要输入的字符串数量。然后,它会循环 n 次,读取每个字符串 s,并调用 test 函数来判断字符串是否符合条件。如果 test 函数返回 1,则输出 "YES",否则输出 "NO"。
test 函数的逻辑如下:
1. 如果字符串中没有字母 'P' 或 'T',则返回 0。
2. 找到字母 'P' 和 'T' 的索引,分别为 x 和 y。
3. 如果 x 大于 y,说明 'P' 在 'T' 的后面,返回 0。
4. 如果 'P' 和 'T' 之间没有字符,返回 0。
5. 将字符串分为三部分:'P' 之前的部分为 b,'P' 和 'T' 之间的部分为 c,'T' 之后的部分为 d。
6. 如果 b、c、d 中包含除字母 A 外的其他字符,则返回 0。
7. 如果 d 等于 b 重复 len(c) 次的结果,则返回 1,否则返回 0。
这段代码可以用来判断一些特定规则的字符串是否符合要求。
相关问题
def test(a): if 'P' not in a or 'T' not in a: # 如果字符串中没有P或者T,则返回0 return 0 x = a.index('P') # 找到P的索引 y = a.index('T') # 找到T的索引 if x > y: # P在T的后面,返回0 return 0 if y == x + 1: # P和T之间没有字符,返回0 return 0 b = a[:x] # P之前的字符串 c = a[x+1:y] # P和T之间的字符串 d = a[y+1:] # T之后的字符串 if not b.isalpha() or not c.isalpha() or not d.isalpha(): # b、c、d中只能包含字母A return 0 if d == b * len(c): # 条件判断 return 1 else: return 0 n = int(input()) for _ in range(n): s = input() if test(s) == 1: print('YES') else: print('NO')
这段代码是一个简单的字符串匹配问题。它接受一个整数n作为输入,表示接下来有n个字符串需要进行匹配。每个字符串s都会通过test函数进行判断,如果满足条件则输出'YES',否则输出'NO'。
test函数首先判断字符串s中是否包含字符'P'和'T',如果不包含则返回0。接着找到字符'P'和字符'T'的索引,如果'P'的索引大于'T'的索引,则返回0。然后,将字符串s分为三部分:P之前的字符串b、P和T之间的字符串c、T之后的字符串d。如果b、c、d中包含非字母字符,则返回0。
最后,如果d等于b重复c长度次数的结果,则返回1,否则返回0。
整个程序循环n次,对每个字符串s进行匹配,并输出结果。
def test(a): x=-1 y=-1 for i in range(len(a)): if a[i]=='P': x=i if a[i]=='T': y=i if x == -1 or y == -1: return 0 if x>y: return 0 if x+1==y: return 0 b=[:x] if x!=0 else[] c=a[x+1:y] d=a[y+1:] if y!=len(a)-1 else[] if not all(i=='A' for i in b): return 0 if not all(i=='A' for i in c): return 0 if not all(i=='A' for i in d): return 0 return 1 if d==b * len(c) else 0 n=input() for i in range(int(n)): s=input() if test(s) == 1: print('YES') else: print('No') 我的代码哪里出了问题
你的代码有几个问题:
1. 在条件判断 `if x+1==y:` 的行末缺少了一个冒号 `:`。
2. 在变量 `b` 的赋值语句中,`b=[:x]` 应该改为 `b=a[:x]`,以获取从开头到 `x` 位置的子字符串。
3. 在条件判断 `return 1 if d==b * len(c) else 0` 的行首多了一个缩进空格,应该与上一行对齐。
4. 最后一个 for 循环缩进不正确,需要将其与前面的代码对齐。
以下是修正后的代码:
```python
def test(a):
x = -1
y = -1
for i in range(len(a)):
if a[i] == 'P':
x = i
if a[i] == 'T':
y = i
if x == -1 or y == -1:
return 0
if x > y:
return 0
if x + 1 == y:
return 0
b = a[:x] if x != 0 else []
c = a[x + 1:y]
d = a[y + 1:] if y != len(a) - 1 else []
if not all(i == 'A' for i in b):
return 0
if not all(i == 'A' for i in c):
return 0
if not all(i == 'A' for i in d):
return 0
return 1 if d == b * len(c) else 0
n = input()
for i in range(int(n)):
s = input()
if test(s) == 1:
print('YES')
else:
print('NO')
```
希望这次能够顺利运行!
阅读全文
相关推荐
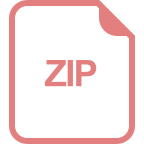
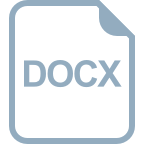
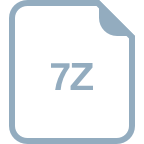
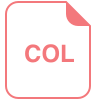
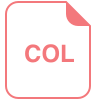
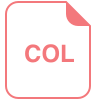
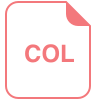
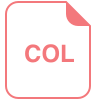
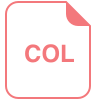
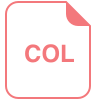







