数据结构c语言 单链表的就地逆置
时间: 2023-10-10 22:03:20 浏览: 125
单链表的就地逆置是指不引入新的节点,只调整原有节点的指针,将单链表逆序排列。
实现单链表的就地逆置可以使用三个指针:prev、curr和next。开始时,将prev指向NULL,curr指向链表的首节点,next指向curr的下一个节点。
接下来,通过循环遍历单链表,每次将curr的指针指向prev,然后将prev、curr和next指针都向后移动一个节点。直到curr指向NULL,表示已经遍历到链表的末尾。
最后,将链表的头节点指向prev,完成就地逆置。
以下是C语言实现的代码示例:
#include <stdio.h>
struct Node {
int data;
struct Node* next;
};
// 单链表就地逆置函数
void reverseList(struct Node** head){
struct Node* prev = NULL;
struct Node* curr = *head;
struct Node* next = NULL;
while(curr != NULL){
next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
*head = prev;
}
// 打印单链表函数
void printList(struct Node* head){
struct Node* temp = head;
while(temp != NULL){
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main(){
// 创建一个示例单链表: 1 -> 2 -> 3 -> 4 -> 5
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
struct Node* fourth = NULL;
struct Node* fifth = NULL;
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
third = (struct Node*)malloc(sizeof(struct Node));
fourth = (struct Node*)malloc(sizeof(struct Node));
fifth = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = fourth;
fourth->data = 4;
fourth->next = fifth;
fifth->data = 5;
fifth->next = NULL;
printf("原始链表: ");
printList(head);
reverseList(&head);
printf("就地逆置后的链表: ");
printList(head);
return 0;
}
运行此程序,输出结果为:
原始链表: 1 2 3 4 5
就地逆置后的链表: 5 4 3 2 1
阅读全文
相关推荐
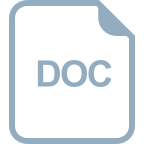
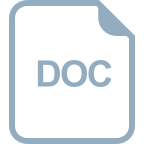
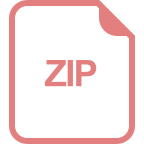
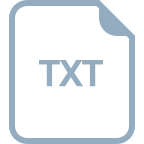



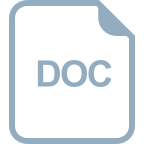
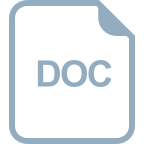
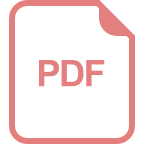
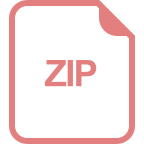
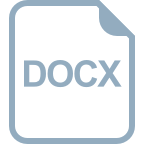
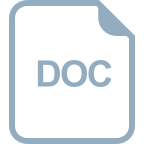
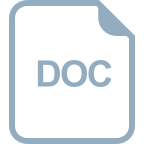
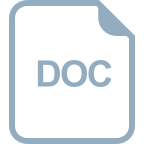