C语言数据结构,尾插法构建单链表,实现删除, 修改,就地逆置操作,编写相应函数完成这些功能
时间: 2024-10-11 09:14:18 浏览: 48
在C语言中,尾插法用于在单链表的末尾高效添加新的元素。以下是创建链表的数据结构及核心函数示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构
struct Node {
int data;
struct Node* next;
};
// 插入节点到链表尾部
void insertAtTail(struct Node** head, int value) {
if (*head == NULL) {
*head = (struct Node*)malloc(sizeof(struct Node));
if (*head == NULL) {
printf("Memory allocation failed.\n");
return;
}
(*head)->data = value;
(*head)->next = NULL;
} else {
struct Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = (struct Node*)malloc(sizeof(struct Node));
if (current->next == NULL) {
printf("Memory allocation failed.\n");
return;
}
current->next->data = value;
current->next->next = NULL;
}
}
// 删除节点(假设我们有一个指向要删除节点的指针prev)
void deleteNode(struct Node** head, struct Node* node) {
if (*head == node) {
*head = node->next;
free(node);
} else {
struct Node* current = *head;
while (current->next != node) {
current = current->next;
}
current->next = node->next;
free(node);
}
}
// 修改节点值(假设我们有一个指向要修改的节点的指针node)
void modifyNode(struct Node** head, int old_value, int new_value) {
struct Node* current = *head;
while (current != NULL && current->data != old_value) {
current = current->next;
}
if (current != NULL) {
current->data = new_value;
} else {
printf("Old value not found in the list.\n");
}
}
// 就地逆置链表
void reverseList(struct Node** head) {
if (*head == NULL || (*head)->next == NULL) {
return;
}
struct Node* prev = NULL;
struct Node* current = *head;
struct Node* nextTemp = NULL;
while (current != NULL) {
nextTemp = current->next;
current->next = prev;
prev = current;
current = nextTemp;
}
*head = prev;
}
// 显示链表
void displayList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
struct Node* head = NULL;
insertAtTail(&head, 1);
insertAtTail(&head, 2);
insertAtTail(&head, 3);
displayList(head);
// 示例删除、修改和逆置操作
deleteNode(&head, head->next); // 删除第二个节点
modifyNode(&head, 2, 4); // 修改第三个节点为4
reverseList(&head); // 就地逆置链表
displayList(head);
return 0;
}
```
这个程序首先创建了一个单链表,然后演示了如何用尾插法添加节点,以及如何通过`deleteNode`、`modifyNode`和`reverseList`函数执行删除、修改和逆置操作。注意在实际应用中,这些操作可能会有边界检查和错误处理。
阅读全文
相关推荐
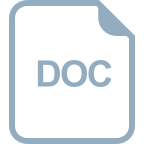
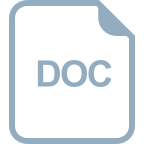
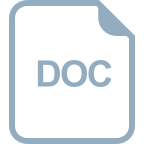















