用C语言实现循环单链表的就地逆置
时间: 2023-03-31 12:02:24 浏览: 141
可以使用以下代码实现循环单链表的就地逆置:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
Node *reverseList(Node *head) {
if (head == NULL || head->next == NULL) {
return head;
}
Node *prev = NULL;
Node *curr = head;
Node *next = head->next;
while (next != head) {
curr->next = prev;
prev = curr;
curr = next;
next = next->next;
}
curr->next = prev;
head->next = curr;
return head;
}
int main() {
Node *head = (Node *)malloc(sizeof(Node));
head->next = head;
Node *tail = head;
for (int i = 1; i <= 5; i++) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = i;
node->next = head;
tail->next = node;
tail = node;
}
printf("Original list: ");
Node *p = head->next;
while (p != head) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
head = reverseList(head);
printf("Reversed list: ");
p = head->next;
while (p != head) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return ;
}
```
这个程序会创建一个循环单链表,然后调用 `reverseList` 函数对其进行就地逆置。最后输出原始链表和逆置后的链表。
阅读全文
相关推荐
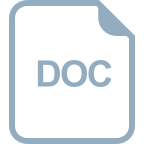
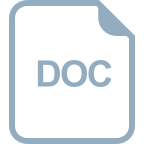
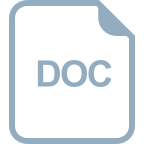

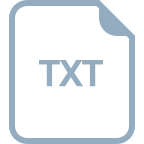








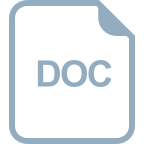
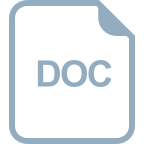


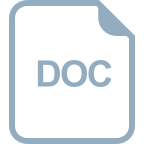
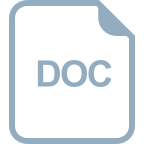