现在有个c++编写的qt程序,有rabbitmq,怎么才能实现在qt程序中加入rabbitmq的接口,实现能够在qt执行出来的界面中,有一个框可以输入文字消息,然后发出,然后另一个框显示接收。使用qamqp。给出详细代码和解释
时间: 2024-11-25 12:17:32 浏览: 42
要在Qt程序中使用QAMQP库来集成RabbitMQ,并提供用户输入发送和接收消息的功能,首先需要安装QAMQP库,并了解它的基本用法。以下是简单的示例代码,以及相应的解释:
```cpp
#include <QApplication>
#include <QQmlEngine>
#include <QQmlContext>
#include "qamqp.h"
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr)
: QMainWindow(parent)
{
qmlEngine()->addImportPath("path_to_qamqp_module"); // 设置QML模块路径,根据实际情况替换
QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
if (!engine.rootObjects().isEmpty()) {
rootObject = engine.rootObjects()[0];
connect(rootObject, &YourQObject::sendMessage, this, &MainWindow::onSendMessage);
connect(rootObject, &YourQObject::receiveMessage, this, &MainWindow::onReceiveMessage);
}
}
private slots:
void onSendMessage(const QString &message) {
YourRabbitMQConnection* rabbitMQConnection = new YourRabbitMQConnection();
auto queueName = ...; // 设置队列名称
rabbitMQConnection->publish(queueName, message.toUtf8()); // 发送消息
delete rabbitMQConnection;
}
void onReceiveMessage(const QString &message) {
qDebug() << "Received message: " << message;
ui->receiverTextBrowser->append(message); // 显示接收到的消息
}
private:
QQmlEngine *qmlEngine;
QObject *rootObject;
signals:
void sendMessage(const QString &message);
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow window;
window.show();
return app.exec();
}
```
在这个例子中:
- `YourQObject`是你的主窗口或者信号槽关联的对象,它需要有`sendMessage`和`receiveMessage`信号和槽用于用户交互。
- `YourRabbitMQConnection`是你自定义的一个类,用于处理RabbitMQ连接、发布和接收消息。你需要实现这个类,包括连接建立、发送消息(`publish`方法)、以及接收消息(通常通过监听事件或回调机制)。
**main.qml** 文件中,可以定义UI元素,如输入框、按钮和文本浏览器,绑定`sendMessage`信号和`receiveMessage`槽。例如:
```qml
import QtQuick 2.15
import QtQuick.Controls 2.15
Window {
id: mainWindow
width: 600
height: 400
title: qsTr("RabbitMQ Chat")
TextField {
id: senderInput
textChanged: parent.sendMessage(senderInput.text)
}
TextBrowser {
id: receiverTextBrowser
}
Row {
anchors.bottom: parent.bottom
Button {
text: "Send"
onClicked: parent.senderInput.clear(); // 清空输入框
signalHandler: parent.sendMessage(senderInput.text)
}
Button {
text: "Quit"
onClicked: app.quit()
}
}
}
```
以上代码展示了基本的用户交互流程,当用户在发送框输入消息并点击"Send"按钮时,会触发`sendMessage`信号,进一步发送到RabbitMQ。接收到的消息则会在接收框中显示。
**相关问题--:**
1. 如何在RabbitMQConnection类中处理连接断开的情况?
2. 如何在QML中显示错误信息,如果RabbitMQ通信失败了怎么办?
3. 如何优雅地处理并发请求,避免消息乱序?
阅读全文
相关推荐

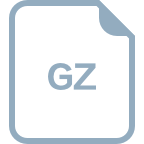












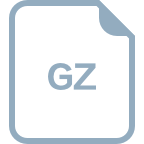


