用c++写一份代码,要求用哈夫曼树进行编码(从终端读入字符集大小n以及n个字符和n个权值,建立赫夫曼树)
时间: 2024-12-25 07:25:08 浏览: 5
在C++中,你可以使用STL(标准模板库)中的`priority_queue`来构建一个最小堆,然后通过递归的方式生成赫夫曼树。这里是一个简单的示例,用于读取字符集大小、字符和权值,构建赫夫曼树,然后生成编码:
```cpp
#include <iostream>
#include <queue>
#include <unordered_map>
struct Node {
char data;
int weight;
int freq;
Node* left = nullptr;
Node* right = nullptr;
// 添加左右孩子节点和更新频率
void add_child(Node* child) {
if (child->freq > 0)
freq += child->freq;
if (left == nullptr)
left = child;
else if (right == nullptr)
right = child;
child->parent = this;
}
};
Node* build_huffman_tree(int n, std::vector<char>& chars, std::vector<int>& weights) {
std::priority_queue<std::pair<int, Node*>, std::vector<std::pair<int, Node*>>, std::greater<int>> huffman_heap;
for (int i = 0; i < n; ++i) {
Node node(chars[i], weights[i], 1);
huffman_heap.push({weights[i], &node});
}
while (huffman_heap.size() > 1) {
Node* left = huffman_heap.top().second;
huffman_heap.pop();
Node* right = huffman_heap.top().second;
huffman_heap.pop();
Node* newNode = new Node;
newNode->add_child(left);
newNode->add_child(right);
huffman_heap.push({left->freq + right->freq, newNode});
}
return huffman_heap.top().second;
}
std::string encode(const std::string& message, const Node* root) {
std::string encoded;
for (char c : message) {
Node* current = root;
while (true) {
encoded += (current->data == c ? '0' : '1');
if (current->left && current->left->data == c)
current = current->left;
else if (current->right && current->right->data == c)
current = current->right;
else
break;
}
}
return encoded;
}
int main() {
int n;
std::cout << "Enter the number of characters: ";
std::cin >> n;
std::vector<char> chars(n);
std::vector<int> weights(n);
std::cout << "Enter the characters and their corresponding weights: ";
for (int i = 0; i < n; ++i) {
std::cin >> chars[i] >> weights[i];
}
Node* huffTree = build_huffman_tree(n, chars, weights);
std::string input;
std::getline(std::cin, input);
std::string encoded = encode(input, huffTree);
std::cout << "Encoded message: " << encoded << "\n";
delete huffTree;
return 0;
}
```
这个程序首先读取字符集大小和权重,然后构建赫夫曼树。接着,用户输入一段文本,程序将其编码。注意,由于Huffman编码是动态生成的,所以实际的编码过程依赖于输入字符集的具体情况。
阅读全文
相关推荐
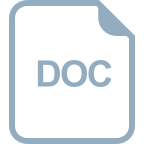
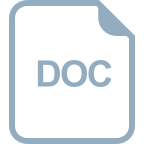
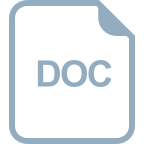

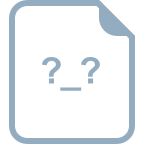
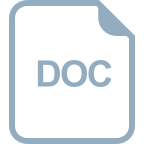
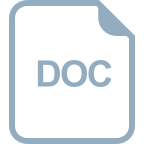
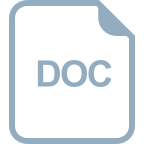
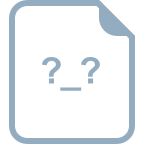
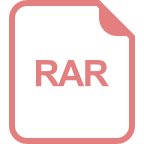
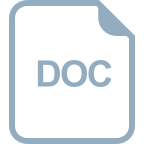
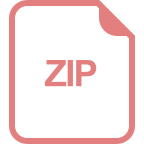
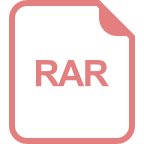
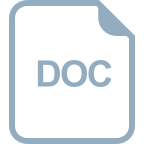
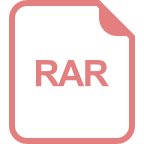
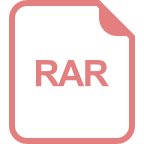
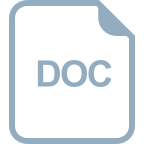
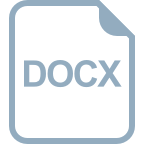