C++红黑树实现Map
时间: 2023-10-22 19:57:00 浏览: 157
当然,我可以帮你实现一个基于红黑树的Map数据结构。下面是一个简单的C++代码示例:
```cpp
#include <iostream>
enum Color { RED, BLACK };
template <typename K, typename V>
struct Node {
K key;
V value;
Color color;
Node* left;
Node* right;
Node* parent;
// 构造函数
Node(const K& k, const V& v, Color c = RED)
: key(k), value(v), color(c), left(nullptr), right(nullptr), parent(nullptr) {}
};
template <typename K, typename V>
class RBTreeMap {
public:
RBTreeMap() : root(nullptr) {}
// 向红黑树中插入键值对
void insert(const K& key, const V& value) {
Node<K, V>* node = new Node<K, V>(key, value);
if (root == nullptr) {
root = node;
node->color = BLACK;
} else {
Node<K, V>* curr = root;
Node<K, V>* parent = nullptr;
while (curr != nullptr) {
parent = curr;
if (key < curr->key)
curr = curr->left;
else if (key > curr->key)
curr = curr->right;
else {
curr->value = value; // 更新值
return;
}
}
node->parent = parent;
if (key < parent->key)
parent->left = node;
else
parent->right = node;
insertFixup(node);
}
}
// 查找指定键的值
V find(const K& key) const {
Node<K, V>* curr = root;
while (curr != nullptr) {
if (key < curr->key)
curr = curr->left;
else if (key > curr->key)
curr = curr->right;
else
return curr->value;
}
return V(); // 未找到,返回默认值
}
private:
Node<K, V>* root;
// 对红黑树进行修复以维持红黑树的性质
void insertFixup(Node<K, V>* node) {
while (node->parent != nullptr && node->parent->color == RED) {
if (node->parent == node->parent->parent->left) {
Node<K, V>* uncle = node->parent->parent->right;
if (uncle != nullptr && uncle->color == RED) {
node->parent->color = BLACK;
uncle->color = BLACK;
node->parent->parent->color = RED;
node = node->parent->parent;
} else {
if (node == node->parent->right) {
node = node->parent;
rotateLeft(node);
}
node->parent->color = BLACK;
node->parent->parent->color = RED;
rotateRight(node->parent->parent);
}
} else {
Node<K, V>* uncle = node->parent->parent->left;
if (uncle != nullptr && uncle->color == RED) {
node->parent->color = BLACK;
uncle->color = BLACK;
node->parent->parent->color = RED;
node = node->parent->parent;
} else {
if (node == node->parent->left) {
node = node->parent;
rotateRight(node);
}
node->parent->color = BLACK;
node->parent->parent->color = RED;
rotateLeft(node->parent->parent);
}
}
}
root->color = BLACK;
}
// 左旋
void rotateLeft(Node<K, V>* node) {
Node<K, V>* rightChild = node->right;
node->right = rightChild->left;
if (rightChild->left != nullptr)
rightChild->left->parent = node;
rightChild->parent = node->parent;
if (node->parent == nullptr)
root = rightChild;
else if (node == node->parent->left)
node->parent->left = rightChild;
else
node->parent->right = rightChild;
rightChild->left = node;
node->parent = rightChild;
}
// 右旋
void rotateRight(Node<K, V>* node) {
Node<K, V>* leftChild = node->left;
node->left = leftChild->right;
if (leftChild->right != nullptr)
leftChild->right->parent = node;
leftChild->parent = node->parent;
if (node->parent == nullptr)
root = leftChild;
else if (node == node->parent->left)
node->parent->left = leftChild;
else
node->parent->right = leftChild;
leftChild->right = node;
node->parent = leftChild;
}
};
int main() {
RBTreeMap<int, std::string> map;
map.insert(1, "one");
map.insert(2, "two");
map.insert(3, "three");
map.insert(4, "four");
std::cout << map.find(2) << std::endl; // 输出: two
std::cout << map.find(5) << std::endl; // 输出: 空字符串(默认值)
return 0;
}
```
这个示例使用红黑树实现了一个简单的Map数据结构。你可以使用`insert`方法向Map中插入键值对,使用`find`方法查找指定键的值。注意,这只是一个简单的实现,还可以根据需要进行扩展和优化。
阅读全文
相关推荐

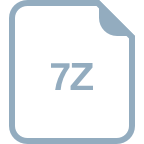
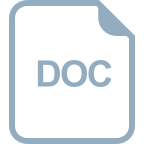
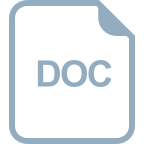
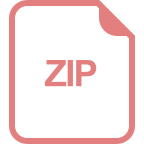
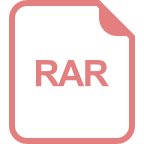
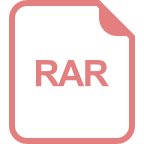
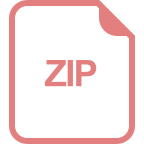
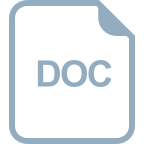
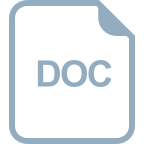
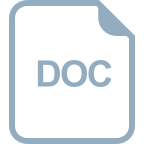
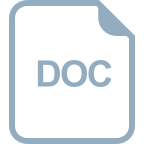

