使用java将geoJson转换成图片且在openlayers中加载
时间: 2024-06-08 09:07:54 浏览: 292
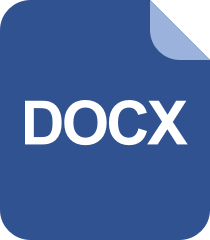
【JavaScript源代码】基于Vue+Openlayer实现动态加载geojson的方法.docx
要将GeoJSON转换为图片并在OpenLayers中加载,可以按照以下步骤进行操作:
1. 在Java中读取GeoJSON文件并将其转换为FeatureCollection对象。可以使用Jackson库来读取和解析GeoJSON文件。
2. 使用Java绘图库(例如Java2D)创建一个图像对象并将FeatureCollection绘制到图像上。您可以使用Feature对象的几何数据来绘制不同的形状。
3. 将图像保存为文件(例如PNG或JPEG格式)。
4. 在OpenLayers中创建一个ImageLayer,并将图像文件的URL设置为图层的源。
以下是Java代码的示例:
```
// 读取GeoJSON文件
ObjectMapper objectMapper = new ObjectMapper();
File file = new File("path/to/geojson/file.json");
FeatureCollection featureCollection = objectMapper.readValue(file, FeatureCollection.class);
// 创建图像对象
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = image.createGraphics();
// 绘制FeatureCollection
for (Feature feature : featureCollection) {
Geometry geometry = feature.getGeometry();
// 根据几何类型绘制不同的形状
if (geometry instanceof Point) {
Point point = (Point) geometry;
g2d.drawOval((int) point.getX(), (int) point.getY(), 10, 10);
} else if (geometry instanceof LineString) {
LineString lineString = (LineString) geometry;
int[] xPoints = new int[lineString.getCoordinates().size()];
int[] yPoints = new int[lineString.getCoordinates().size()];
for (int i = 0; i < lineString.getCoordinates().size(); i++) {
xPoints[i] = (int) lineString.getCoordinates().get(i).getX();
yPoints[i] = (int) lineString.getCoordinates().get(i).getY();
}
g2d.drawPolyline(xPoints, yPoints, lineString.getCoordinates().size());
} else if (geometry instanceof Polygon) {
Polygon polygon = (Polygon) geometry;
int[] xPoints = new int[polygon.getExteriorRing().getCoordinates().size()];
int[] yPoints = new int[polygon.getExteriorRing().getCoordinates().size()];
for (int i = 0; i < polygon.getExteriorRing().getCoordinates().size(); i++) {
xPoints[i] = (int) polygon.getExteriorRing().getCoordinates().get(i).getX();
yPoints[i] = (int) polygon.getExteriorRing().getCoordinates().get(i).getY();
}
g2d.drawPolygon(xPoints, yPoints, polygon.getExteriorRing().getCoordinates().size());
}
}
// 保存图像到文件
File output = new File("path/to/image/file.png");
ImageIO.write(image, "png", output);
// 在OpenLayers中创建ImageLayer
ImageLayer imageLayer = new ImageLayer();
imageLayer.setSource(new ImageStatic("path/to/image/file.png", null, extent, pixelRatio));
map.addLayer(imageLayer);
```
请注意,此示例中的代码仅用于演示目的,并且可能需要进行修改以适应您的具体情况。
阅读全文
相关推荐
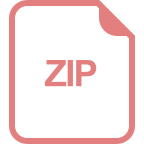
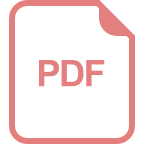
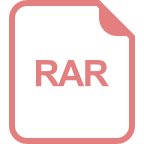
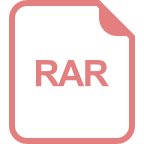
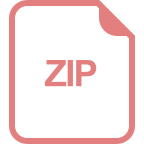
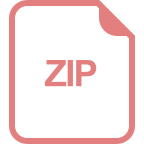
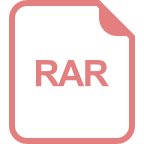
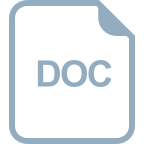
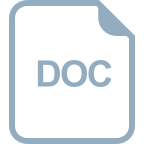
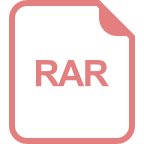
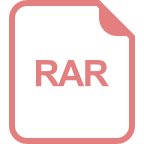
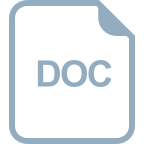
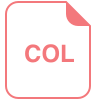
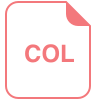


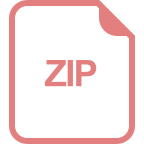
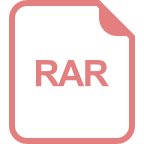
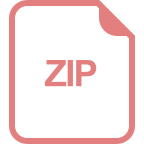