在Linux编写C代码或者汇编代码,操作串口,网卡,音卡等设备,至少操作两种设备,给出代码
时间: 2024-01-02 09:05:12 浏览: 265
好的,这个问题需要一些准备工作才能回答。首先,我们需要安装适当的开发工具和驱动程序,以便能够操作串口、网卡和音卡设备。其次,需要一些基础知识,如C语言编程和硬件编程等。
以下是一个简单的C语言程序,用于打开和写入串口设备(假设使用的是/dev/ttyS0设备):
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios tty;
char buffer[20] = "Hello, world!";
fd = open("/dev/ttyS0", O_RDWR);
if (fd == -1) {
printf("Failed to open device.\n");
return -1;
}
tcgetattr(fd, &tty);
cfsetospeed(&tty, B9600);
cfsetispeed(&tty, B9600);
tty.c_cflag |= (CLOCAL | CREAD);
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8;
tty.c_cflag &= ~PARENB;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CRTSCTS;
tty.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
tty.c_iflag &= ~(IXON | IXOFF | IXANY);
tty.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &tty);
write(fd, buffer, sizeof(buffer));
close(fd);
return 0;
}
```
这个程序使用了Linux系统提供的串口设备驱动程序,通过打开设备文件并设置一些参数,然后使用write()函数向设备写入数据。其中,tcgetattr()和tcsetattr()函数用于获取和设置串口设备的属性,cfsetospeed()和cfsetispeed()函数用于设置串口的波特率。
下面是一个简单的汇编程序,用于读取网卡设备(假设使用的是eth0设备):
```asm
section .data
buffer db 1024
section .text
global _start
_start:
; open device
mov eax, 5
mov ebx, eth0
mov ecx, O_RDONLY
int 0x80
cmp eax, 0
jl error
mov ebx, eax
; read from device
mov eax, 3
mov ecx, buffer
mov edx, 1024
int 0x80
cmp eax, 0
jl error
; print data
mov eax, 4
mov ebx, 1
mov ecx, buffer
mov edx, eax
int 0x80
; close device
mov eax, 6
mov ebx, [ebp-4]
int 0x80
; exit
mov eax, 1
xor ebx, ebx
int 0x80
error:
; handle error
mov eax, 1
mov ebx, 2
int 0x80
```
这个程序使用了Linux系统提供的网络设备驱动程序,通过系统调用实现了打开、读取和关闭设备的功能。其中,int 0x80是一个系统调用指令,eax寄存器用于指定调用的功能号,ebx、ecx、edx寄存器用于传递参数和返回值。
最后是一个简单的C语言程序,用于播放音频文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
int main()
{
int rc;
snd_pcm_t *handle;
snd_pcm_hw_params_t *params;
snd_pcm_uframes_t frames;
char *buffer;
int size, rate, channels;
size = 8192;
buffer = (char *)malloc(size);
rc = snd_pcm_open(&handle, "default", SND_PCM_STREAM_PLAYBACK, 0);
if (rc < 0) {
printf("Failed to open device.\n");
return -1;
}
snd_pcm_hw_params_alloca(¶ms);
snd_pcm_hw_params_any(handle, params);
snd_pcm_hw_params_set_access(handle, params, SND_PCM_ACCESS_RW_INTERLEAVED);
snd_pcm_hw_params_set_format(handle, params, SND_PCM_FORMAT_S16_LE);
snd_pcm_hw_params_set_channels(handle, params, 2);
snd_pcm_hw_params_set_rate_near(handle, params, &rate, 0);
snd_pcm_hw_params_set_period_size_near(handle, params, &frames, 0);
snd_pcm_hw_params(handle, params);
while (1) {
rc = fread(buffer, 1, size, stdin);
if (rc == 0) {
break;
} else if (rc != size) {
memset(buffer + rc, 0, size - rc);
}
snd_pcm_writei(handle, buffer, frames);
}
snd_pcm_drain(handle);
snd_pcm_close(handle);
free(buffer);
return 0;
}
```
这个程序使用了Linux系统提供的音频设备驱动程序,通过snd_pcm_open()和snd_pcm_writei()函数实现了打开、写入和关闭设备的功能。其中,snd_pcm_hw_params_set_*()函数用于设置音频设备的属性,snd_pcm_hw_params()函数用于应用这些属性。这个程序从标准输入读取音频数据,并通过snd_pcm_writei()函数写入音频设备。snd_pcm_drain()函数用于等待所有数据播放完毕。
阅读全文
相关推荐
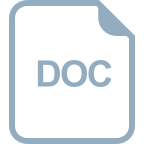
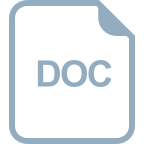
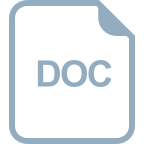
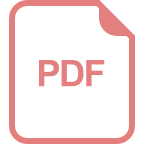
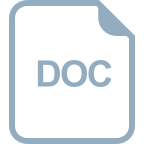
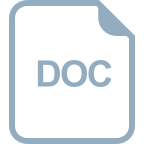
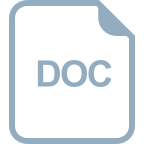
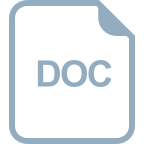
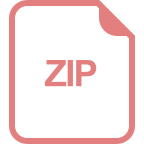
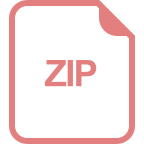
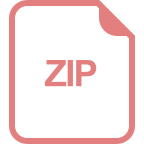
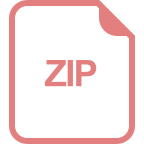
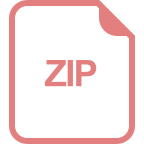
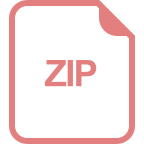
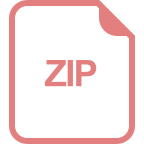