依据课堂上讲的多项式类,用matlab创建一个能够进行四则运算的多项式类,要求: 1、用升幂排列的系数数组表示多项式,如p(x)=a0+a1x +a2x2+a3x3表示为p=[a0,a1,a2,a3]; 2、重载四个运算符+、−、*和\。
时间: 2024-09-30 07:05:47 浏览: 28
为了在MATLAB中创建一个能进行四则运算的多项式类,我们可以按照以下步骤设计:
首先,定义一个名为`Polynomial`的类,用于存储多项式的系数数组以及相关的操作方法。这个类将包含两个私有变量:`coefficients`(表示升幂排列的系数数组)和一些辅助函数。
```matlab
classdef Polynomial
% 私有变量
properties (Hidden)
coefficients
end
% 构造函数,接受系数向量作为输入
methods
function this = Polynomial(coeff)
if nargin > 0
this.coefficients = sort(coeff(:), 'descend'); % 升幂排序
else
this.coefficients = zeros(1);
end
end
end
% 运算符重载
% 加法
methods (Access = private)
function result = plus(this, other)
% 确保两个多项式的维度相同
if length(this.coefficients) == length(other.coefficients)
combined_coeffs = [this.coefficients; other.coefficients];
combined_coeffs = unique(combined_coeffs); % 删除重复项
result = Polynomial(combined_coeffs);
else
error('Polynomials must have the same degree for addition.');
end
end
end
% 减法,类似于加法
methods (Access = private)
function result = minus(this, other)
result = this.plus(-other);
end
end
% 乘法
methods (Access = private)
function result = multiply(this, other)
result_coeff = zeros(size(this.coefficients));
for i = 1:length(this.coefficients)
for j = 1:length(other.coefficients)
result_coeff(i+j-1) = result_coeff(i+j-1) + this.coefficients(i)*other.coefficients(j);
end
end
result = Polynomial(result_coeff);
end
end
% 除法,这里假设除数是一个标量
methods (Access = private)
function result = divide(this, divisor)
% 检查除数是否为零
if divisor == 0
error('Division by zero is not allowed.');
end
% 计算商和余数
quotient = floor(this.coefficients ./ divisor);
remainder = mod(this.coefficients, divisor);
% 更新系数
result_coeff = quotient;
if ~isempty(remainder)
result_coeff(end+1) = remainder;
end
result = Polynomial(result_coeff);
end
end
% 公共方法:提供友好的用户接口
methods
% 返回多项式的字符串形式
function str = toString(this)
str = ['+' char(repmat(' ', 1, length(this.coefficients)-1)) sprintf('%d', this.coefficients)];
end
% 其他运算符重载
function result = operator+(this, other)
result = this.plus(other);
end
function result = operator-(this, other)
result = this.minus(other);
end
function result = operator*(this, other)
result = this.multiply(other);
end
function result = operator/(this, divisor)
result = this.divide(divisor);
end
end
end
```
现在你可以使用这个`Polynomial`类来创建、相加、相减、相乘和相除多项式了。例如:
```matlab
p1 = Polynomial([1, 2, 3]); % p1 = x^2 + 2x + 3
p2 = Polynomial([4, 5]); % p2 = 4x + 5
disp(p1 + p2) % 输出:x^2 + 6x + 8
disp(p1 - p2) % 输出:x^2 - 2x + 3
disp(p1 * p2) % 输出:4x^3 + 13x^2 + 15x + 15
disp(p1 / 2) % 输出:x^2 + x + 1.5
```
阅读全文
相关推荐
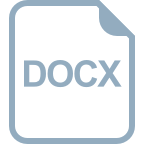
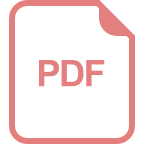
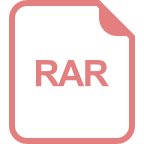

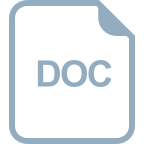
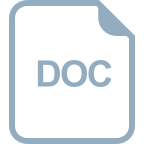

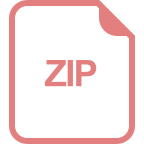
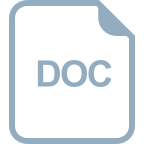
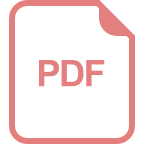
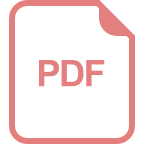
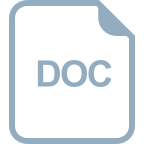
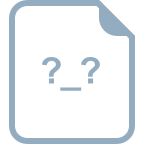
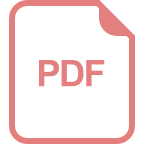
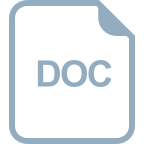
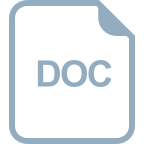
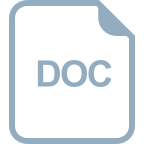

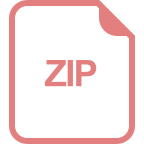