设计一个算法,通过一趟遍历确定长度为n的单链表中值最大的节点,返回该节点的数据域。用c语言编程
时间: 2024-09-11 16:17:22 浏览: 53
设计这样一个算法,我们需要遍历整个链表一次,同时记录下我们遇到的最大值。以下是这个算法的步骤和C语言实现的代码示例:
步骤:
1. 定义一个指向链表首节点的指针,并初始化为链表头节点的地址。
2. 初始化一个变量,用于存储遍历过程中遇到的最大值,该变量可设置为链表头节点的数据值。
3. 遍历链表,对于每个节点,比较其数据域与当前已知的最大值,如果当前节点的数据更大,则更新最大值变量。
4. 继续遍历,直到链表尾部(即下一个节点为NULL)。
5. 遍历结束后,返回最大值变量。
C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int data;
struct ListNode *next;
};
// 函数声明
int findMaxInLinkedList(struct ListNode *head);
int main() {
// 示例:创建一个链表并找到最大值
// 这里仅是示例,实际使用时需要根据实际情况创建和初始化链表
struct ListNode *head = malloc(sizeof(struct ListNode));
head->data = 1;
head->next = malloc(sizeof(struct ListNode));
head->next->data = 3;
head->next->next = malloc(sizeof(struct ListNode));
head->next->next->data = 2;
head->next->next->next = NULL;
int max = findMaxInLinkedList(head);
printf("链表中的最大值为:%d\n", max);
// 释放链表内存
struct ListNode *current = head;
while (current != NULL) {
struct ListNode *next = current->next;
free(current);
current = next;
}
return 0;
}
// 查找单链表中最大值的函数实现
int findMaxInLinkedList(struct ListNode *head) {
if (head == NULL) {
return -1; // 空链表返回-1或者合适的错误码
}
int max = head->data; // 假设第一个节点的值最大
struct ListNode *current = head->next; // 从第二个节点开始遍历
while (current != NULL) {
if (current->data > max) {
max = current->data; // 更新最大值
}
current = current->next;
}
return max; // 返回最大值
}
```
阅读全文
相关推荐
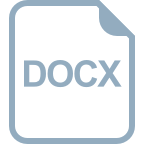
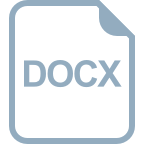
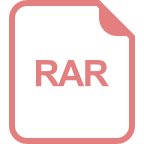








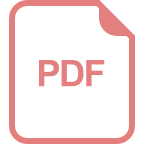
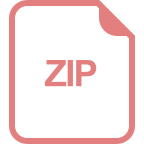
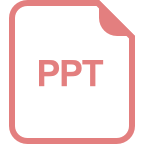
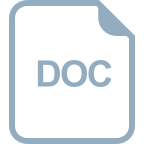
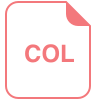
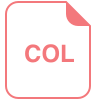

