Linux文件的写入,用write写入数字0~300
时间: 2023-06-02 17:02:26 浏览: 174
可以使用以下命令将数字0~300写入文件中:
```
for i in {0..300}; do echo $i >> file.txt; done
```
其中,`{0..300}` 表示从0到300的数字范围,`echo $i` 将每个数字输出到屏幕上,并使用 `>>` 符号将其写入文件 `file.txt` 中。
相关问题
Linux中使用C语言对文件的写入,用write写入数字0~300
下面是一个示例程序,使用C语言在Linux中对文件进行写入操作,写入数字0到300:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd = open("output.txt", O_WRONLY | O_CREAT, 0666);
if (fd < 0) {
perror("failed to open file");
exit(1);
}
char buf[4];
int i;
for (i = 0; i <= 300; i++) {
sprintf(buf, "%d", i);
write(fd, buf, sizeof(buf));
}
close(fd);
return 0;
}
```
该程序首先通过`open`函数打开一个名为`output.txt`的文件,如果文件不存在则创建该文件,并设置文件权限为0666。`O_WRONLY`表示以只写方式打开文件。
接着,程序使用一个循环,从0到300依次写入每个数字。使用`sprintf`函数将数字转换为字符串,然后使用`write`函数将字符串写入文件中,写入操作的长度为`sizeof(buf)`,即为4字节,因为每个数字最多只占4个字符。
最后,程序使用`close`函数关闭文件,并返回0表示程序正常结束。
linux写入文件命令
在Linux中,写入文件的常用命令是"write"和"echo"。
使用"write"命令可以将指定的内容写入到指定用户的终端。其语法为:
write [用户名 [终端 [文件名]
使用"echo"命令可以将指定的内容写入到指定的文件中。其语法为:
echo [内容 > [文件名]
另外,你还可以使用文件IO函数来在C语言中进行文件写入操作。例如,可以使用open函数打开文件,使用write函数将内容写入文件,最后使用close函数关闭文件。下面是一个示例代码:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
int main() {
int fd;
char *content = "file write";
fd = open("./file1", O_RDWR);
if (fd == -1) {
printf("open file1 failed\n");
fd = open("./file1", O_RDWR | O_CREAT, 0600);
if (fd > 0) {
printf("create file1 successed\n");
}
}
printf("open file1 successed, fd=%d\n", fd);
write(fd, content, strlen(content));
close(fd);
return 0;
}
请注意,在C语言中,使用文件IO函数进行文件写入操作时,需要包含相应的头文件,并在写入完成后关闭文件。
阅读全文
相关推荐
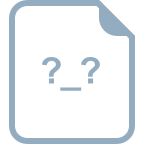
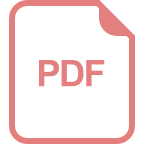
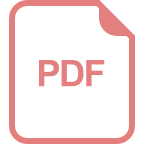
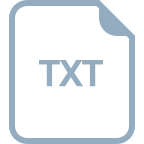
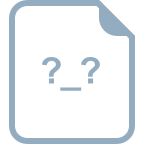
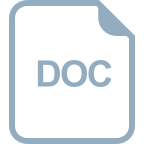
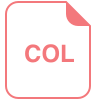








