使用C语言在Linux系统中对文件进行读写操作. 补全write_File函数,完成向文件写入字符串功能。并返回实际写入字符个数。 补全readLine函数,完成从文件中读取一行的功能(不包括换行符),并返回实际读取的字符个数(文件的换行符号为\n)。
时间: 2024-02-27 14:52:09 浏览: 36
下面是使用 C 语言在 Linux 系统中对文件进行读写操作的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024 // 定义每行最大长度
// 向文件中写入字符串,返回实际写入的字符个数
int write_File(const char *filename, const char *str) {
FILE *fp;
int len;
fp = fopen(filename, "w"); // 以写入方式打开文件
if (fp == NULL) {
printf("Error opening file %s for writing.\n", filename);
return -1;
}
len = strlen(str);
if (fwrite(str, sizeof(char), len, fp) != len) { // 写入字符串
printf("Error writing to file %s.\n", filename);
return -1;
}
fclose(fp); // 关闭文件
return len;
}
// 从文件中读取一行,返回实际读取的字符个数
int readLine(const char *filename, char *line) {
FILE *fp;
int len = 0;
char ch;
fp = fopen(filename, "r"); // 以只读方式打开文件
if (fp == NULL) {
printf("Error opening file %s for reading.\n", filename);
return -1;
}
while ((ch = fgetc(fp)) != EOF && ch != '\n') { // 逐个字符读取一行
line[len++] = ch;
}
line[len] = '\0';
fclose(fp); // 关闭文件
return len;
}
int main() {
char *filename = "test.txt";
char *str = "Hello, world!";
char line[MAX_LINE_LEN];
// 向文件中写入字符串
int write_len = write_File(filename, str);
printf("Write %d characters to file %s.\n", write_len, filename);
// 从文件中读取一行
int read_len = readLine(filename, line);
printf("Read %d characters from file %s: %s\n", read_len, filename, line);
return 0;
}
```
在 `write_File` 函数中,使用 `fopen` 函数打开文件,然后使用 `fwrite` 函数将字符串写入文件,最后使用 `fclose` 函数关闭文件。
在 `readLine` 函数中,使用 `fopen` 函数打开文件,然后使用 `fgetc` 函数逐个字符读取一行,直到遇到换行符或文件结束符。最后使用 `fclose` 函数关闭文件。
相关推荐
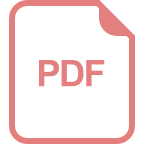
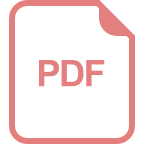
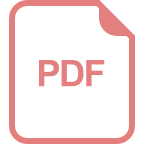











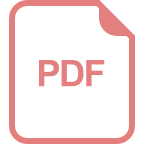
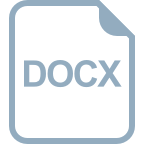
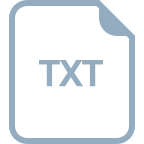
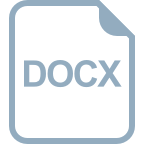