系统调用编程: 1. 使用系统调用open read write,完成一个C语言程序:该程序接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “学号 MYFILE”,其中学号填为自己的学号。 2. 改造上面的程序,完成: i. 使用strict alternation,并利用该程序生成2个进程,这两个进程写同一个文件,要求:a.互斥写,即只有一个进程写完后,才能让另一个进程写; b. 一个进程写入内容:“自己学号的后3位PROC1 MYFILE1”;另一个进程写入内容:“自己学号的后3位PROC2 MYFILE2
时间: 2024-02-12 11:06:07 浏览: 77
1. 下面是使用系统调用open read write完成的C语言程序,可以实现对指定文件的读写:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
char buf[100];
int fd, n;
if (argc != 3) {
printf("Usage: %s <filename> <r/w>\n", argv[0]);
exit(1);
}
if ((fd = open(argv[1], (argv[2][0] == 'r') ? O_RDONLY : O_WRONLY | O_CREAT, 0644)) == -1) {
perror("open");
exit(1);
}
if (argv[2][0] == 'r') {
if ((n = read(fd, buf, sizeof(buf))) == -1) {
perror("read");
exit(1);
}
printf("Read %d bytes from file %s:\n%s\n", n, argv[1], buf);
} else {
sprintf(buf, "%s %s", "学号", "MYFILE");
if ((n = write(fd, buf, strlen(buf))) == -1) {
perror("write");
exit(1);
}
printf("Write %d bytes to file %s:\n%s\n", n, argv[1], buf);
}
close(fd);
return 0;
}
```
该程序接收两个参数,参数1为要读写的文件名,参数2为标志位,用于指定是读还是写。如果标志位为'r',则读取文件内容并打印;如果标志位为'w',则向文件中写入一个字符串。
2. 下面是使用strict alternation实现的多进程程序,可以实现两个进程互斥地向同一个文件中写入不同的内容:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/wait.h>
#define FILENAME "file.txt"
#define FILESIZE 100
static void proc1(char *buf, int fd) {
sprintf(buf, "%s %s PROC1 MYFILE1", "学号", "MYFILE");
lseek(fd, 0, SEEK_SET);
if (write(fd, buf, strlen(buf)) == -1) {
perror("write");
exit(1);
}
}
static void proc2(char *buf, int fd) {
sprintf(buf, "%s %s PROC2 MYFILE2", "学号", "MYFILE");
lseek(fd, 0, SEEK_SET);
if (write(fd, buf, strlen(buf)) == -1) {
perror("write");
exit(1);
}
}
int main() {
char buf[FILESIZE];
int fd, i;
pid_t pid;
if ((fd = open(FILENAME, O_WRONLY | O_CREAT | O_TRUNC, 0644)) == -1) {
perror("open");
exit(1);
}
for (i = 0; i < 2; i++) {
if ((pid = fork()) == -1) {
perror("fork");
exit(1);
} else if (pid == 0) {
if (i == 0) {
proc1(buf, fd);
} else {
proc2(buf, fd);
}
exit(0);
}
}
for (i = 0; i < 2; i++) {
wait(NULL);
}
close(fd);
return 0;
}
```
该程序使用了fork函数生成两个子进程,每个子进程都会执行不同的函数(proc1或proc2),向同一个文件中写入不同的内容。由于使用了strict alternation机制,两个进程会交替执行,保证了互斥写的效果。注意,在写文件之前,需要将文件指针移动到文件开头。
阅读全文
相关推荐
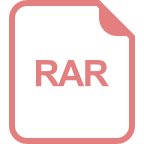
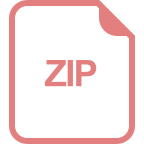






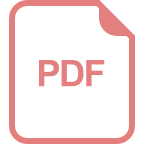
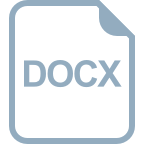
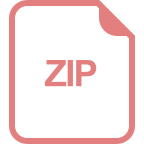
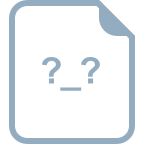
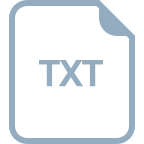
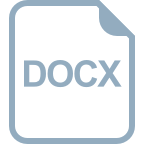
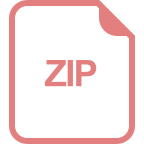