linux使用系统调用open read write,完成一个C语言程序:该程序接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “20009200666 MYFILE”
时间: 2024-02-20 17:01:36 浏览: 22
好的,下面是一个示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
if (argc != 3) { // 两个参数: 文件名和读写标志
printf("Usage: %s <file> <r/w>\n", argv[0]);
return 1;
}
int fd = open(argv[1], argv[2][0] == 'r' ? O_RDONLY : O_WRONLY | O_CREAT, 0644); // 根据读写标志打开文件
if (fd < 0) {
printf("Failed to open file %s\n", argv[1]);
return 1;
}
char buf[1024];
ssize_t bytes;
if (argv[2][0] == 'r') { // 读取文件内容
bytes = read(fd, buf, sizeof(buf));
if (bytes < 0) {
printf("Failed to read file %s\n", argv[1]);
close(fd);
return 1;
}
printf("%s\n", buf);
} else { // 写入内容到文件
bytes = write(fd, "20009200666 MYFILE", 18);
if (bytes < 0) {
printf("Failed to write to file %s\n", argv[1]);
close(fd);
return 1;
}
}
close(fd);
return 0;
}
```
使用方法:
- 编译程序: `gcc -o file_rw file_rw.c`
- 读取文件: `./file_rw myfile r`
- 写入文件: `./file_rw myfile w`
相关推荐
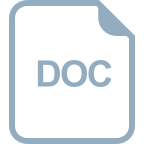
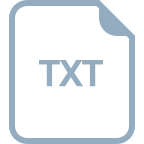
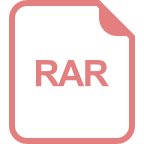







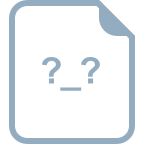
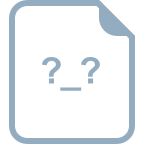
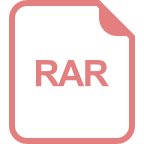
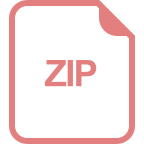
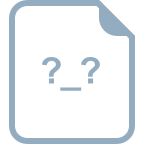