使用C语言,调用Linux的文件系统接口函数open、read、write、close,编写一个读写文本文件的程序,并将文本内容使用printf打印到终端。
时间: 2024-10-07 07:06:30 浏览: 76
在C语言中,为了操作Linux文件系统,你可以使用头文件`unistd.h`和`fcntl.h`,它们包含了基本的文件操作接口。下面是一个简单的示例程序,它会打开一个文本文件(假设名为"example.txt"),读取内容,然后写入新的内容并关闭文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#define FILE_PATH "example.txt"
int main() {
int file_descriptor; // 文件描述符
char* content = "这是新的内容"; // 要写入的内容
ssize_t bytes_read;
size_t bytes_written;
// 打开文件,O_RDWR表示读写模式,O_CREAT如果文件不存在则创建,O_TRUNC表示清空现有文件
if ((file_descriptor = open(FILE_PATH, O_RDWR | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR)) == -1) {
perror("Error opening file");
return 1;
}
// 写入文件
bytes_written = write(file_descriptor, content, strlen(content));
if (bytes_written != strlen(content)) {
perror("Error writing to file");
close(file_descriptor);
return 1;
}
// 读取文件内容
char buffer[1024]; // 假设最多能读取1KB
bytes_read = read(file_descriptor, buffer, sizeof(buffer));
if (bytes_read > 0) {
printf("原始内容:\n%s", buffer); // 打印读取的内容
} else {
perror("Error reading from file");
}
// 关闭文件
if (close(file_descriptor) == -1) {
perror("Error closing file");
}
return 0;
}
```
在这个程序中,我们首先尝试打开文件,然后写入新内容,接着读取文件内容并将其打印出来,最后关闭文件。注意,这个例子假设文件权限允许我们进行读写操作,如果权限不足可能会导致错误。
阅读全文
相关推荐

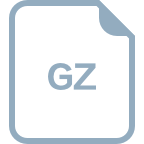















