使用系统调用open read write,完成一个C语言程序:该程序接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “学号 MYFILE”,其中学号填为自己的学号。
时间: 2024-03-25 15:35:54 浏览: 109
以下是一个使用系统调用 `open`、`read`、`write` 实现的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
int fd, flags;
char buffer[BUFFER_SIZE];
size_t bytes;
if (argc != 3) {
fprintf(stderr, "Usage: %s <filename> <flags>\n", argv[0]);
exit(EXIT_FAILURE);
}
// 打开文件
fd = open(argv[1], O_RDWR);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
flags = atoi(argv[2]);
if (flags == 0) {
// 读取文件
bytes = read(fd, buffer, BUFFER_SIZE);
if (bytes == -1) {
perror("read");
exit(EXIT_FAILURE);
}
printf("Read %zu bytes: %s\n", bytes, buffer);
} else {
// 写入文件
char *content = malloc(BUFFER_SIZE);
sprintf(content, "学号 %s", "MYFILE"); // 替换学号为自己的学号
bytes = write(fd, content, strlen(content));
if (bytes == -1) {
perror("write");
exit(EXIT_FAILURE);
}
printf("Write %zu bytes: %s\n", bytes, content);
free(content);
}
// 关闭文件
if (close(fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
return 0;
}
```
该程序接收两个参数:文件名和标志位。标志位为0表示读取文件,为1表示写入文件。
在读取文件时,程序会使用 `read` 函数读取文件内容,并打印读取到的数据。
在写入文件时,程序会先使用 `malloc` 函数动态分配内存,然后使用 `sprintf` 函数将字符串 "学号 MYFILE" 中的 "MYFILE" 替换为自己的学号,最后使用 `write` 函数写入文件。
请注意,该程序只适用于文本文件,如果要读写二进制文件,请使用 `open` 函数时指定 `O_BINARY` 标志位。同时,程序中没有对文件权限进行控制,请谨慎使用。
阅读全文
相关推荐
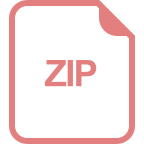







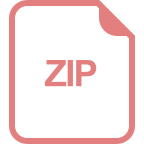
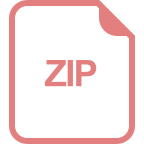
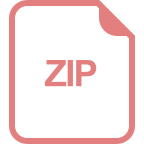
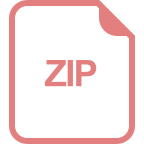
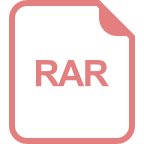
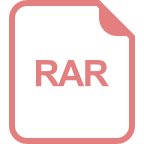